- Sphere Engine overview
- Compilers
- Overview
- API
- Widgets
- Resources
- Problems
- Overview
- API
- Widgets
- Handbook
- Resources
- Containers
- Overview
- Glossary
- API
- Workspaces
- Handbook
- Resources
- RESOURCES
- Programming languages
- Modules comparison
- Webhooks
- Infrastructure management
- API changelog
- FAQ
Sphere Engine Problems API offers the ability to manage and use programming problems for the automated verification of programming skills in processes such as recruitment, education, e-learning, onboarding or training.
When creating a programming problem, you can specify:
- description of the problem,
- assessment type (e.g., maximization, minimization),
- test cases (i.e., data used to test solutions), consisting of:
- input data,
- reference output data,
- judge program (the component responsible for determining the correctness of the test case solution),
- master judge program (the component responsible for the final evaluation of the correctness of the solution).
When submitting a solution to a problem we can specify:
- the source code of the program,
- the programming language.
Sphere Engine Problems API offers the ability to execute programs in dozens of programming languages, including C++, C#, Go, Haskell, Java, Kotlin, Node.js, PHP, Python, Ruby, Scala, or Swift among others (full list of supported programming languages).
Data available after the process of evaluating the solution includes:
- the verdict regarding the correctness of the solution,
- score (in the case of optimization problems or problems assessed partially),
- execution time,
- memory consumption,
- data streams containing:
- generated output data,
- runtime error,
- warnings and compilation errors,
- additional information returned by the judge programs.
For a full list of retrievable data please refer to the Sphere Engine Problems API Docs.
If a submission meets all the requirements of your challenge, it will show up as "accepted" in the system. Otherwise, you can check what the reasons were for its failure.
There are five possible statuses of a submission:
- Accepted - the program is executed within the required time limit and the answer is consistent with the pre-defined solution,
- Wrong answer - the program is executed correctly, but the answer is not consistent with the pre-defined solution,
- Time limit exceeded - the execution time exceeds the imposed time limit. Possible causes of this status include bugs in the submitted code, such as an unforeseen infinite loop, or a solution which is far from optimal and runs too slowly,
- Runtime error - an error appears during execution, which causes the process to stop. One of the reasons could be a memory or stack overflow error in a programming language such as C,
- Compilation error - an error which causes the submitted source code to not compile correctly.
Important: To successfully use Sphere Engine Problems API you need to have a working Sphere Engine account with a dedicated API endpoint and API token. Register for a Sphere Engine account by filling in the sign-up form.
Web service
All URLs referring to the Sphere Engine Problems API have a common base: https://<customer_id>.problems.sphere-engine.com/api/v4/
called an
endpoint. We authorize users using an access token which should be given as a parameter named access_token for
every use of the API method. The management of access tokens is available in Sphere Engine client panel
API Tokens section.
For example, the test method available at a relative address /test
should be executed using the following address
along with the access token: https://<customer_id>.problems.sphere-engine.com/api/v4/test?access_token=<access_token>
.
The Sphere Engine Problems API is compliant with the REST architecture. This results in the following characteristics of the service:
- every API use is an
HTTP
request, - the
HTTP
request method specifies the type of the API method:GET
– retrieving data,POST
– creating a new resource,PUT
– updating the data of the resource,DELETE
– deleting the resource,
- The
HTTP
response code informs about the execution of the API method:- codes beginning with 2 (e.g.,
200
,201
) inform about success, - codes starting with 4 (e.g.,
404
) inform about the unsuccessful execution of the method (e.g., referring to a non-existent resource), - codes beginning with 5 (e.g.,
500
) inform about connection or server errors.
- codes beginning with 2 (e.g.,
Requests
Transfer of data to the API is done using HTTP
parameters. Therefore, any client library that supports the HTTP
protocol can be used to integrate with the Sphere Engine service. Most modern application production technologies offer
a wide range of solutions enabling HTTP
communication. You can also find solutions dedicated to supporting RESTful
API services.
For GET
and DELETE
requests, parameters should be included in the query, i.e., as part of the URL. For example:
https://<customer_id>.problems.sphere-engine.com/api/v4/submissions?access_token=<access_token>&ids=40,41,42
.
POST
and PUT
requests allow for sending parameters in three formats:
- JSON (i.e., Content-Type: application/json),
- FORM (i.e., Content-Type: application/x-www-form-urlencoded),
- MULTIPART (i.e., Content-Type: multipart/form-data).
Read on for a detailed description of parameters available for every API method.
Response format
The API response is an HTTP
response. It consists of HTTP
status and message body.
The REST
architecture uses HTTP
response codes to summarize the execution of the API method. The table below lists
the response codes together with their interpretation.
Response code | Interpretation |
---|---|
200 | success in GET , PUT and DELETE methods |
201 | success in POST methods |
400 | incorrect request (e.g., incorrect parameter format) |
401 | authentication failed (i.e., missing or incorrect access token) |
403 | lack of authorization to access the resource |
404 | the requested resource does not exist |
5xx | a family of errors occurring server-side or connection-side |
The response is always returned in the JSON
format (i.e., Content-type: application/json). In case of an API method
execution error (i.e., 4**
response code), the structure of the response is as follows:
{
"error_code": xxx,
"message": "message describing the error"
}
The error_code
field contains an integer identifying the error and allowing for its easy interpretation. The detailed
description of the API methods contains a list of errors along with their corresponding numerical values which appear in
the error_code
field.
Note: The HTTP
response code and the error code (i.e., the error_code
field) are two different response
components.
Submission status
The programs sent to be executed using the Sphere Engine system are called submissions. A submission consists of the source code of the program, the programming language and (optionally) the input data to be processed by the program.
The response of the API method for retrieving the data of submission (i.e., GET /submissions/:id
) contains two fields
defining the current status of the submission.
The binary executing
field helps you quickly verify if the submission is being processed by the system. This is
determined by the values:
true
- the submission is still being processed by the system. At this point, the data generated by the program is not yet available,false
- at this point, the submission has already left the part of the Sphere Engine system responsible for the execution of programs. All information (e.g., execution time, memory consumption, data streams) has been established and is available.
The integer field called status
precisely determines the status of the submission. Values should be interpreted by
the following tables:
List of transient states (i.e., executing = true
)
Status | Name | Description |
---|---|---|
0 | waiting | submission is waiting in the queue |
1,2 | compilation | program is being compiled (for compiled languages) |
3 | execution | program is being executed |
4 | compiling judge | compilation of the judge (for judges implemented in compiled languages) |
5 | executing judge | execution of the judge program |
6 | compiling master judge | compilation of the master judge (for judges implemented in compiled languages) |
7 | executing master judge | execution of the master judge program |
8 | testing program | the program is being tested |
List of final states (i.e., executing = false
)
Status | Name | Description |
---|---|---|
11 | compilation error | the program could not be executed due to a compilation error |
12 | runtime error | an error occurred while the program was running (e.g., division by zero) |
13 | time limit exceeded | the program exceeded the time limit |
14 | wrong answer | incorrect solution |
15 | accepted | correct solution |
17 | memory limit exceeded | the program exceeded the memory limit |
19 | illegal system call | the program tried to call illegal system function |
20 | internal error | an unexpected error occurred on the Sphere Engine side try making the submission again and if this occurs again, please contact us |
21 | judge error | test case judge or master judge error |
22 | problem error | problem configuration error |
Waiting for the submission to be executed
Note: To get faster results and bypass polling we recommend using the webhook integration.
After creating a new submission in the system, some time is required for it to be executed. A recommended practice while waiting for the submission to finish processing is to implement the following scenario:
- Create a submission using the
POST /submissions
method, - Wait 2 seconds,
- Retrieve submission data using the
GET /submissions/:id
method, - [optional] Present the current status of the submission to the user,
- If executing = true, go back to step /2/,
- [optional] Present the data of the submission to the user.
The diagram below presents the concept of this scenario. The duration shown in the diagram is hypothetical:
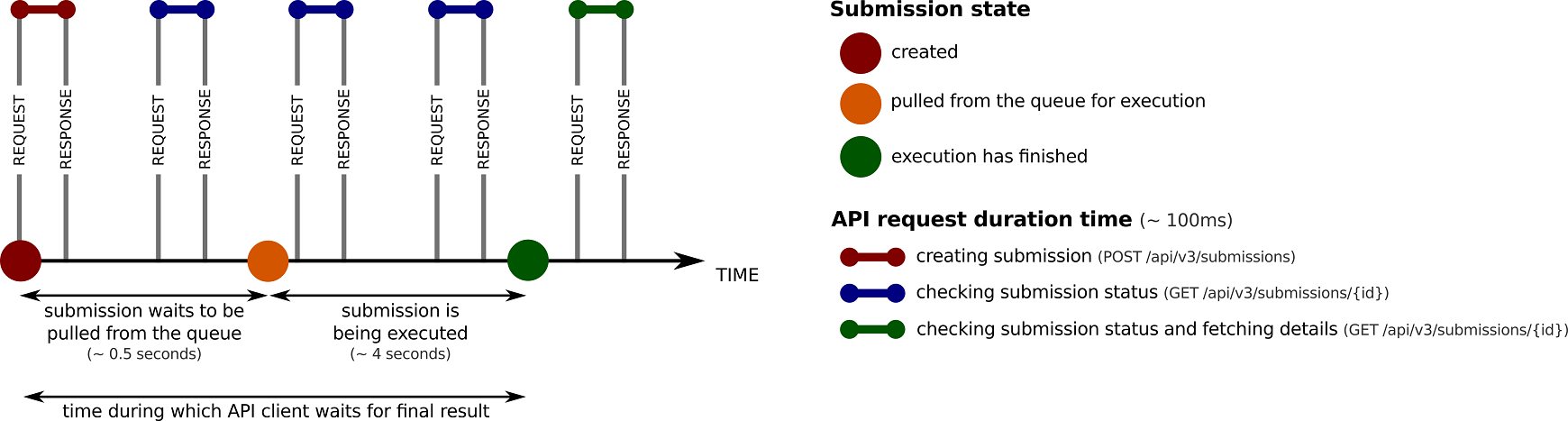
Important: Intensive server polling (i.e., no time interval between requests) while waiting for a submission to be processed can lead to a reaction from the Sphere Engine API security system. High intensity of API requests can be interpreted as a DoS/DDoS attack. In this case, Sphere Engine servers may refuse further access to the service.
Submission verification time
The time it takes Sphere Engine Problems API to process a submission is directly related to the set of test cases (i.e., their number and time limits used).
It should be noted that the use of high time limits can lead to prolonged waiting for the result of the submission. Long
waiting time affects both the user experience and system load. For this reason, you should maintain a timeout of
1
second, unless the change is justified.
This formula to estimate the worst-case scenario processing time of a submission may be helpful:
T = N(ti + b)
, where:
N
– number of test cases,ti
– time limit of the i-th test case,b
– fixed time overhead associated with running the program.
Calculating verification time example
Let us consider a programming problem with 10 test cases (N = 10
), each with a time limit of 6
seconds (i.e.,
ti = 6
for each test case). In many situations (e.g., for erroneous programs that never stop
running) execution time will significantly exceed 60 seconds (i.e., T = N(ti + b) = 10(6 + b) = 60
+ 10b > 60
seconds).
Client libraries
There are client libraries available for the following programming languages:
In addition, there are also examples of using the Sphere Engine API for several other technologies:
For each API
method below, there is an example of calling this method from the client library or using selected
technologies.
Migration from previous versions
Due to extensive changes that were made when creating version 4 of the Sphere Engine API, we were unable to maintain compatibility with its previous version.
However, the migration process to the current version shouldn't be troublesome. From the user's perspective, most of the changes are related to the structure of the parameters of the API methods and the structure of the response.
Refer to the full list of changes for details.
API methods
Testing Problems API. Every successful usage returns identical response.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
message | string | message that states that it's possible to use API |
Examples
curl -X GET 'https://<endpoint>/api/v4/test?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful test() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->test();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful test() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.test()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/test?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // test message in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class test {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/test?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/test?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"message": "You can use Sphere Engine Problems API."
}
Returns a list of supported compilers.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | compiler id |
items[].name | string | compiler name |
items[].short | string | short name |
items[].versions[].id | integer | compiler version id |
items[].versions[].name | string | compiler version name |
items[].highlighters | object | syntax highlighters |
items[].highlighters.ace | string | syntax highlighting identifier for Ace |
items[].highlighters.geshi | string | syntax highlighting identifier for Geshi |
items[].highlighters.pygments | string | syntax highlighting identifier for Pygments |
items[].highlighters.highlights | string | syntax highlighting identifier for Highlights |
items[].highlighters.rouge | string | syntax highlighting identifier for Rouge |
items[].highlighters.codemirror | string | syntax highlighting identifier for CodeMirror |
items[].highlighters.highlightjs | string | syntax highlighting identifier for highlight.js |
items[].highlighters.prism | string | syntax highlighting identifier for Prism |
items[].highlighters.monaco | string | syntax highlighting identifier for Monaco Editor |
items[].highlighters.code_prettify | string | syntax highlighting identifier for Google Code Prettify |
Examples
curl -X GET 'https://<endpoint>/api/v4/compilers?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful getCompilers() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getCompilers();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful compilers() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.compilers()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/compilers?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of compilers in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getCompilers {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/compilers?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/compilers?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"items": [
{
"id": 1,
"name": "C++",
"short": "CPP",
"versions": [
{
"id": 1,
"name": "gcc 6.3"
}
],
"highlighters": {
"ace": "c_cpp",
"geshi": "cpp",
"pygments": "cpp",
"highlights": "c",
"rouge": "cpp",
"codemirror": "clike",
"highlightjs": "cpp",
"prism": "cpp",
"monaco": "cpp",
"code_prettify": "cpp"
}
},
{
"id": 116,
"name": "Python 3",
"short": "PYTHON3",
"versions": [
{
"id": 1,
"name": "3.5"
}
],
"highlighters": {
"ace": "python",
"geshi": "python",
"pygments": "python",
"highlights": "python",
"rouge": "python",
"codemirror": "python",
"highlightjs": "python",
"prism": "python",
"monaco": "python",
"code_prettify": "python"
}
}
]
}
Creates a new submission. You can create a submission with many files using multi-file mode. Every usage of this method decreases submissions pool for your account.
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
problemId |
form | integer | - |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
source |
form | string | - | source code (see also: multi-file mode) |
compilerId |
form | integer | - | compiler id |
compilerVersionId | form | integer | 1 | compiler version identifier |
tests | form | string | <as in problem settings> | comma separated list of test case numbers to be executed against submission |
files | form | array | <no files> | set of files that form a project to be executed (see also: multi-file mode) |
executionMode | form | string | <as in problem settings> |
execution mode fast | isolated |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
402 | 2 | inactive account or empty submissions poll |
400 | 201304 | source not provided |
400 | 200204 | problem not provided |
400 | 200201 | problem not found |
400 | 200202 | access to problem denied |
400 | 201101 | compiler not found |
400 | 201201 | compiler version not found |
400 | 201104 | compiler not provided |
400 | 201108 | compiler not available |
400 | 201306 | source size limit exceeded |
400 | 205205 | invalid format of test cases sequence |
400 | 205206 | invalid test cases sequence |
400 | 205209 | empty test cases sequence |
400 | 205405 | invalid execution mode |
400 | 1003 | creation failed |
Success Response
Field | Type | Description |
---|---|---|
id | integer | id of created submission |
Examples
$ cat request.json
{
"problemId": 42,
"compilerId": 1,
"source": "<source code>"
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/submissions?access_token=<access_token>'
<?php
/**
* Example presents error handling for createSubmission() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 42;
$source = '<source code>';
$compiler = 1;
try {
$response = $client->createSubmission($problemId, $source, $compiler);
// response['id'] stores the ID of the created submission
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 402) {
echo 'Unable to create submission';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.create() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
source = '<source code>'
nonexistingCompiler = 99999
try:
response = client.submissions.create(problemId, source, nonexistingCompiler)
# response['id'] stores the ID of the created submission
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 402:
print('Unable to create submission')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionData = {
problemId: 42,
compilerId: 11,
source: '<source_code>'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions?access_token=' + accessToken,
method: 'POST',
form: submissionData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 402) {
console.log('Unable to create submission');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class createSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient ();
// define request parameters
NameValueCollection formData = new NameValueCollection ();
formData.Add("problemId", "42");
formData.Add("source", "<source_code>");
formData.Add("compilerId", "11");
// send request
byte[] responseBytes = client.UploadValues ("https://" + endpoint + "/api/v4/submissions?access_token=" + accessToken, "POST", formData);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse)response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
} else if(statusCode == HttpStatusCode.PaymentRequired) {
Console.WriteLine("Unable to create submission");
} else if(statusCode == HttpStatusCode.BadRequest) {
StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.UTF8);
Console.WriteLine(reader.ReadToEnd());
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_data = {
"problemId" => "42",
"compilerId" => "11",
"source" => '<source_code>'
};
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/submissions?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri);
request.set_form_data(submission_data)
begin
response = http.request(request)
# process response
case response
when Net::HTTPCreated
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
when Net::HTTPPaymentRequired
puts "Unable to create submission"
when Net::HTTPBadRequest
body = JSON.parse(response.body)
puts "Error code: " + body["error_code"].to_s + ", details available in the message: " + body["message"].to_s
end
rescue => e
puts "Connection error"
end
Response example
{
"id": 42
}
Returns information about submissions. Results are sorted ascending by id.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
ids |
query | string |
comma separated list of submission identifiers max: 20 |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
400 | 205104 | identifiers are not provided |
400 | 205105 | invalid format of identifiers |
400 | 205106 | maximum number of identifiers exceeded |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | submission id |
items[].executing | boolean | indicates whether submission is being executed |
items[].date | string |
date and time of submission creation [yyyy-mm-dd hh:mm:ss TZD] note that server time is used |
items[].executionMode | string |
execution mode fast | isolated |
items[].compiler | object | submission compiler |
items[].compiler.id | integer | compiler id |
items[].compiler.name | string | compiler name |
items[].compiler.version | object | compiler version |
items[].compiler.version.id | integer | compiler version id |
items[].compiler.version.name | string | compiler version name |
items[].problem | object | problem details |
items[].problem.id | integer | problem id |
items[].problem.code | string | [deprecated] problem code |
items[].problem.name | string | problem name |
items[].problem.uri | string | link to problem details |
items[].result | object | submission result |
items[].result.status | object | submission status |
items[].result.status.code | integer |
status code see section Submission status |
items[].result.status.name | string | status name |
items[].result.score | float | final score |
items[].result.time | float | execution time [seconds] |
items[].result.memory | integer | memory consumed by the program [kilobytes] |
items[].result.signal | integer | signal raised by the program |
items[].result.signal_desc | string | description of the raised signal |
items[].result.testcases[].number | integer | test case number |
items[].result.testcases[].status | object | test case status |
items[].result.testcases[].status.code | integer |
test case status code see section Submission status |
items[].result.testcases[].status.name | string | test case status name |
items[].result.testcases[].score | float | test case score |
items[].result.testcases[].time | float | test case execution time [seconds] |
items[].result.testcases[].memory | integer | test case memory consumed by the program [kilobytes] |
items[].result.testcases[].signal | integer | test case signal raised by the program |
items[].result.testcases[].signal_desc | string | description of the raised test case signal |
items[].uri | string | link to submission details |
Examples
curl -X GET \
-H 'Content-Type: application/json' \
'https://<endpoint>/api/v4/submissions?ids=:ids&access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmissions() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmissions(array(2017, 2018));
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.getMulti() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.getMulti([2017, 2018])
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionsIds = [2017, 2018];
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions?ids=' + submissionsIds.join() + '&access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of submissions in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"items": [
{
"id": 42,
"executing": false,
"date": "2017-02-24 10:02:26 +00:00",
"executionMode": "fast",
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 6.3"
}
},
"problem": {
"id": 142,
"code": "EXPRBL",
"name": "Example Problem",
"uri": "https://<endpoint>/api/v4/problems/142?access_token=<access_token>"
},
"result": {
"status": {
"code": 15,
"name": "accepted"
},
"score": 100,
"time": 1.24,
"memory": 2048,
"signal": 0,
"signal_desc": "",
"testcases": [
{
"number": 0,
"status": {
"code": 15,
"name": "accepted"
},
"score": 100,
"memory": 2048,
"time": 1.24,
"signal": 0,
"signal_desc": ""
}
]
},
"uri": "https://<endpoint>/api/v4/submissions/42?access_token=<access_token>"
}
]
}
Returns information about a submission.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | string | submission id |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | submission not found |
Success Response
Field | Type | Description |
---|---|---|
id | integer | submission id |
executing | boolean | indicates whether submission is being executed |
date | string |
date and time of submission creation [yyyy-mm-dd hh:mm:ss TZD] note that server time is used |
executionMode | string |
execution mode fast | isolated |
compiler | object | submission compiler |
compiler.id | integer | compiler id |
compiler.name | string | compiler name |
compiler.version | object | compiler version |
compiler.version.id | integer | compiler version id |
compiler.version.name | string | compiler version name |
problem | object | problem details |
problem.id | integer | problem id |
problem.code | string | [deprecated] problem code |
problem.name | string | problem name |
problem.uri | string | link to problem details |
result | object | submission result |
result.status | object | submission status |
result.status.code | integer |
status code see section Submission status |
result.status.name | string | status name |
result.score | float | final score |
result.time | float | execution time [seconds] |
result.memory | integer | memory consumed by the program [kilobytes] |
result.signal | integer | signal raised by the program |
result.signal_desc | string | description of the raised signal |
result.streams | object | submission streams |
result.streams.source | object | source code |
result.streams.source.size | integer | source code length [bytes] |
result.streams.source.uri | string | link to the file with source code |
result.streams.output | object | output data |
result.streams.output.size | integer | size of output data [bytes] |
result.streams.output.uri | string | link to the file with output data |
result.streams.error | object | error data |
result.streams.error.size | integer | size of error data [bytes] |
result.streams.error.uri | string | link to the file with error data |
result.streams.cmpinfo | object | compilation info |
result.streams.cmpinfo.size | integer | size of compilation data [bytes] |
result.streams.cmpinfo.uri | string | link to the file with compilation data |
result.streams.debug | object | debug data |
result.streams.debug.size | integer | size of debug data [bytes] |
result.streams.debug.uri | string | link to the file with debug data |
result.testcases[].number | integer | test case number |
result.testcases[].status | object | test case status |
result.testcases[].status.code | integer |
test case status code see section Submission status |
result.testcases[].status.name | string | test case status name |
result.testcases[].score | float | test case score |
result.testcases[].time | float | test case execution time [seconds] |
result.testcases[].memory | integer | test case memory consumed by the program [kilobytes] |
result.testcases[].signal | integer | test case signal raised by the program |
result.testcases[].signal_desc | string | description of the raised test case signal |
Examples
curl -X GET \
-H 'Content-Type: application/json' \
'https://<endpoint>/api/v4/submissions/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmission() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmission(2017);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the submission is forbidden';
}elseif ($e->getCode() == 404) {
echo 'Submission does not exist';
}
}
"""
Example presents error handling for submissions.get() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.get(2017)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the submission is forbidden')
elif e.code == 404:
print('Submission does not exist')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 2017;
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions/' + submissionId + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Submision not found');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
// define request parameters
int submissionId = 2017;
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/submissions/" + submissionId + "?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
} else if (statusCode == HttpStatusCode.Forbidden) {
Console.WriteLine("Access denied");
} else if (statusCode == HttpStatusCode.NotFound) {
Console.WriteLine("Submission not found");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_id = "2017"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/submissions/" + submission_id + "?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
when Net::HTTPForbidden
puts "Access denied"
when Net::HTTPNotFound
puts "Submission not found"
end
rescue => e
puts "Connection error"
end
Response example
{
"id": 42,
"executing": false,
"date": "2017-02-24 10:02:26 +00:00",
"executionMode": "isolated",
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 6.3"
}
},
"problem": {
"id": 142,
"code": "EXPRBL",
"name": "Example Problem",
"uri": "https://<endpoint>/api/v4/problems/142?access_token=<access_token>"
},
"result": {
"status": {
"code": 15,
"name": "accepted"
},
"score": 100,
"time": 1.24,
"memory": 2048,
"signal": 0,
"signal_desc": "",
"streams": {
"source": {
"size": 189,
"uri": "https://<endpoint>/api/v4/submissions/42/source-42?access_token=<access_token>"
},
"output": {
"size": 11,
"uri": "https://<endpoint>/api/v4/submissions/42/stdout-42?access_token=<access_token>"
},
"error": null,
"cmpinfo": null,
"debug": {
"size": 96,
"uri": "https://<endpoint>/api/v4/submissions/42/stdout-42?access_token=<access_token>"
}
},
"testcases": [
{
"number": 0,
"status": {
"code": 15,
"name": "accepted"
},
"score": 100,
"memory": 2048,
"time": 1.24,
"signal": 0,
"signal_desc": ""
}
]
}
}
Returns raw stream data.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | submission id |
stream |
path | string |
stream name enum: source, output, error, cmpinfo or debug |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | submission not found |
404 | 1101 | stream not found |
400 | 1103 | reading stream failed |
Examples
curl -X GET 'https://<endpoint>/api/v4/submissions/:id/:stream?access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmissionFile() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmissionFile(2017, 'source');
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the submission is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for judges.getJudgeFile() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.getSubmissionFile(2017, 'source')
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the submission is forbidden')
elif e.code == 404:
print('Non existing resource, error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 1;
var stream = 'source'
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions/' + submissionId + '/' + stream + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(response.body); // raw data from selected stream
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
Raw data from selected stream.
Creates a new problem
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
code | form | string | <generated> | [deprecated] problem code; you can set it manually, however, you need to handle a case when the code is already taken |
name |
form | string | - | problem name |
body | form | string | <empty> | problem description |
typeId | form | integer | 0 (binary) |
problem type enum:
|
masterjudgeId |
form | integer | - | master judge id |
interactive | form | boolean | false | interactive problem flag |
executionMode | form | string | isolated |
execution mode fast | isolated |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
400 | 202309 | empty problem code |
400 | 202404 | problem name not provided |
400 | 202409 | empty problem name |
400 | 202308 | problem code is not unique |
400 | 202305 | problem code is invalid |
400 | 202105 | problem type is incorrect |
400 | 202205 | judge type is incorrect |
400 | 200404 | master judge not provided |
400 | 200402 | access to master judge denied |
400 | 200401 | master judge not found |
400 | 205405 | invalid execution mode |
400 | 1003 | creation failed |
Success Response
Field | Type | Description |
---|---|---|
id | integer | id of created problem |
code | string | [deprecated] code of created problem |
Examples
$ cat request.json
{
"name": "Example problem",
"masterjudgeId": 1001
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/problems?access_token=<access_token>'
<?php
/**
* Example presents error handling for createProblem() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$name = "Example problem";
$masterjudgeId = 1001;
try {
$response = $client->createProblem($name, $masterjudgeId);
// response['id'] stores the ID of the created problem
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for problems.create() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
name = 'Example problem'
try:
response = client.problems.create(name)
# response['id'] stores the ID of the created problem
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemData = {
name: 'Example',
masterjudgeId: 1001
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems?access_token=' + accessToken,
method: 'POST',
form: problemData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // problem data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"id": 43,
"code": "PROBLEMCODE"
}
Returns the list of problems of the quantity given by limit parameter starting from the offset parameter.
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
limit | query | integer | 10 |
limit of problems to get max: 100 |
offset | query | integer | 0 | offset |
shortBody | query | boolean | false | whether shortened body should be returned |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | problem id |
items[].code | string | [deprecated] problem code |
items[].name | string | problem name |
items[].shortBody | string |
shortened problem description if shortBody = false, then null |
items[].lastModified | object | |
items[].lastModified.body | integer | timestamp of the last modification date of problem name or problem description |
items[].lastModified.settings | integer | timestamp of the last modification date of problem settings (type, interactivity, master judge, active test cases, new test cases, updated test cases) |
items[].permissions | object | |
items[].permissions.edit | boolean | true if you can edit this problem |
items[].permissions.clone | boolean | true if you can clone this problem |
items[].executionMode | string |
execution mode fast | isolated |
items[].uri | string | link to problem details |
paging | object | |
paging.limit | integer | items per page |
paging.offset | integer | first item |
paging.total | integer | total number of items |
paging.pages | integer | total number of pages for the current limit |
paging.prev | string | previous page, link or null if no page |
paging.next | string | next page, link or null if no page |
Examples
curl -X GET 'https://<endpoint>/api/v4/problems?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful getProblems() API method
*/
use SphereEngine\Api\ProblemsClientV4;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$response = $client->getProblems();
"""
Example presents usage of the successful problems.all() API method
"""
from sphere_engine import ProblemsClientV4
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
response = client.problems.all()
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of problems in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
Response example
{
"items": [
{
"id": 8,
"code": "EXPRBL",
"name": "Example Problem",
"shortBody": null,
"lastModified": {
"body": 1486478234,
"settings": 1487929707
},
"permissions": {
"edit": true,
"clone": true
},
"uri": "https://<endpoint>/api/v4/problems/8?access_token=<access_token>"
},
{
"id": 9,
"code": "ANOPRBL",
"name": "Another Problem",
"shortBody": null,
"lastModified": {
"body": 1486491532,
"settings": 1486496511
},
"permissions": {
"edit": true,
"clone": true
},
"executionMode": "fast",
"uri": "https://<endpoint>/api/v4/problems/9?access_token=<access_token>"
}
],
"paging": {
"limit": 2,
"offset": 4,
"total": 7,
"pages": 4,
"prev": "https://<endpoint>/api/v4/problems?offset=2&access_token=<access_token>",
"next": "https://<endpoint>/api/v4/problems?offset=6&access_token=<access_token>"
}
}
Retrieves an existing problem
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
id |
path | integer | - |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
shortBody | query | boolean | false | whether shortened body should be returned |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
Success Response
Field | Type | Description |
---|---|---|
id | integer | problem id |
code | string | [deprecated] problem code |
name | string | problem name |
shortBody | string |
shortened problem description if shortBody = false, then null |
body | string | problem description |
type | object | |
type.id | integer |
problem type id
|
type.name | string |
problem type name
|
interactive | boolean | interactive problem flag |
masterjudge | object | |
masterjudge.id | integer | master judge id |
masterjudge.name | string | master judge name |
masterjudge.uri | string | master judge uri |
testcases[].number | integer | test case number |
testcases[].active | boolean | if test is active |
lastModified | object | |
lastModified.body | integer | timestamp of the last modification date of problem name or problem description |
lastModified.settings | integer | timestamp of the last modification date of problem settings (type, interactivity, master judge, active test cases, new test cases, updated test cases) |
executionMode | string |
execution mode fast | isolated |
permissions | object | |
permissions.edit | boolean | true if you can edit this problem |
permissions.clone | boolean | true if you can clone this problem |
Examples
curl -X GET 'https://<endpoint>/api/v4/problems/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for getProblem() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 987654321;
try {
$response = $client->getProblem($problemId);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Problem does not exist';
}
}
"""
Example presents error handling for problems.get() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
try:
response = client.problems.get(problemId)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Problem does not exist')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // problem data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Problem not found');
}
}
}
});
Response example
{
"id": 43,
"code": "EXPRBL",
"name": "Example Problem",
"shortBody": null,
"body": "problem description",
"type": {
"id": 0,
"name": "binary"
},
"interactive": false,
"masterjudge": {
"id": 1001,
"name": "Score is % of correctly solved sets",
"uri": "https://<endpoint>/api/v4/judges/1001?access_token=<access_token>"
},
"testcases": [
{
"number": 0,
"active": true
},
{
"number": 1,
"active": false
}
],
"lastModified": {
"body": 1486478234,
"settings": 1487929707
},
"executionMode": "fast",
"permissions": {
"edit": true,
"clone": true
}
}
Updates an existing problem
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
name | form | string | problem name |
body | form | string | problem description |
typeId | form | integer |
problem type enum:
|
interactive | form | boolean | interactive problem flag |
masterjudgeId | form | integer | master judge id |
activeTestcases | form | string | list of active test cases ids (comma separated) |
executionMode | form | string |
execution mode fast | isolated |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
400 | 202409 | empty problem name |
400 | 202105 | problem type is incorrect |
400 | 202205 | judge type is incorrect |
400 | 200402 | access to master judge denied |
400 | 200401 | master judge not found |
400 | 205205 | invalid format of test cases sequence |
400 | 205206 | invalid test cases sequence |
400 | 205405 | invalid execution mode |
400 | 1003 | update failed |
Examples
$ cat request.json
{
"name": "<new_name>"
}
$ curl -X PUT \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/problems/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for updateProblem() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 42;
$newProblemName = 'New example problem name';
try {
$response = $client->updateProblem($problemId, $newProblemName);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Problem does not exist';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for problems.update() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
newProblemName = 'New example problem name'
try:
response = client.problems.update(problemId, newProblemName)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Problem does not exist')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
var problemData = {
name: 'New name'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '?access_token=' + accessToken,
method: 'PUT',
form: problemData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log('Problem updated');
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Problem does not exist');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{}
Deletes an existing problem
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
400 | 1003 | delete failed |
Examples
curl -X DELETE 'https://<endpoint>/api/v4/problems/:id?access_token=<access_token>'
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '?access_token=' + accessToken,
method: 'DELETE'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log('Problem deleted');
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Problem not found');
}
}
}
});
Response example
{}
Creates a problem test case
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
id |
path | integer | - |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
input | form | string | <empty> | input data |
output | form | string | <empty> | output data |
timeLimit | form | float | 1 | time limit [seconds] |
judgeId |
form | integer | - | judge id |
active | form | boolean | true | whether test case should be active |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
400 | 200304 | judge not provided |
400 | 200302 | access to judge denied |
400 | 200301 | judge not found |
400 | 202205 | judge type is incorrect |
400 | 201905 | invalid format of time limit |
400 | 205306 | maximum number of test cases exceeded |
400 | 1103 | creation failed |
Success Response
Field | Type | Description |
---|---|---|
number | integer | number of created test case |
Examples
$ cat request.json
{
"input": "input content",
"timeLimit": 10,
"output": "output content",
"judgeId": 1
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/problems/:id/testcases?access_token=<access_token>'
<?php
/**
* Example presents error handling for createProblemTestcase() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$id = 42;
$input = "model input";
$output = "model output";
$timeLimit = 5;
$judgeId = 1;
$active = true;
try {
$response = $client->createProblemTestcase($id, $input, $output, $timeLimit, $judgeId, $active);
// response['number'] stores the number of created testcase
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Problem does not exist';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for createProblemTestcase() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
id = 42
input = 'model input'
output = 'model output'
time_limit = 5
judgeId = 1
try:
response = client.problems.createTestcase(id, input, output, time_limit, judgeId)
# response['number'] stores the number of created testcase
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Problem does not exist')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
var testcaseData = {
input: 'Input',
output: 'Output',
timelimit: 5,
judgeId: 1
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '/testcases?access_token=' + accessToken,
method: 'POST',
form: testcaseData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // testcase data in JSON
}
else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Problem does not exist');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"number": 3
}
Retrieves a list of problem test cases
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
Success Response
Field | Type | Description |
---|---|---|
items[].number | integer | test case number |
items[].active | boolean | indicates whether test case is active |
items[].limits | object | |
items[].limits.time | float | time limit [seconds] |
items[].judge | object | |
items[].judge.id | integer | judge id |
items[].judge.name | string | judge name |
items[].judge.uri | string | link to judge |
items[].uri | string | link to test case details |
Examples
curl -X GET 'https://<endpoint>/api/v4/problems/:id/testcases?access_token=<access_token>'
<?php
/**
* Example presents error handling for getProblemTestcases() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 987654321;
try {
$response = $client->getProblemTestcases($problemId);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Problem does not exist';
}
}
"""
Example presents error handling for problems.allTestcases() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
try:
response = client.problems.allTestcases(problemId)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Problem does not exist')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '/testcases?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of testcases in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Problem not found');
}
}
}
});
Response example
{
"items": [
{
"number": 0,
"active": true,
"limits": {
"time": 1
},
"judge": {
"id": 1,
"name": "Ignores extra whitespaces",
"uri": "https://<endpoint>/api/v4/judges/1?access_token=<access_token>"
},
"uri": "https://<endpoint>/api/v4/problems/1/testcases/0?access_token=<access_token>"
},
{
"number": 1,
"active": false,
"limits": {
"time": 0.8
},
"judge": {
"id": 1,
"name": "Ignores extra whitespaces",
"uri": "https://<endpoint>/api/v4/judges/1?access_token=<access_token>"
},
"uri": "https://<endpoint>/api/v4/problems/1/testcases/1?access_token=<access_token>"
}
]
}
Retrieves problem test case
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
number |
path | integer | test case number |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
404 | 1101 | testcase not found |
Success Response
Field | Type | Description |
---|---|---|
number | integer | test case number |
active | boolean | indicates whether test case is active |
input | object | |
input.size | integer | size of input data [bytes] |
input.uri | string | link to input data |
output | object | |
output.size | integer | size of output data [bytes] |
output.uri | string | link to output data |
limits | object | |
limits.time | float | time limit [seconds] |
judge | object | |
judge.id | integer | judge id |
judge.name | string | judge name |
judge.uri | string | link to judge |
Examples
curl -X GET 'https://<endpoint>/api/v4/problems/:id/testcases/:number?access_token=<access_token>'
<?php
/**
* Example presents error handling for getProblemTestcase() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 42;
$testcaseNumber = 0;
try {
$response = $client->getProblemTestcase($problemId, $testcaseNumber);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for problems.getTestcase() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
nonexistingTestcaseNumber = 999
try:
response = client.problems.getTestcase(problemId, nonexistingTestcaseNumber)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
# aggregates two possible reasons of 404 error
# non existing problem or testcase
print('Non existing resource (problem, testcase), details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
var testcaseNumber = 0;
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '/testcases/' + testcaseNumber + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // testcase data in JSON
}
else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"number": 0,
"active": true,
"input": {
"size": 7,
"uri": "https://<endpoint>/api/v3/problems/1/testcases/0/input-EXPRBL-0.txt?access_token=<access_token>"
},
"output": {
"size": 9,
"uri": "https://<endpoint>/api/v3/problems/1/testcases/0/output-EXPRBL-0.txt?access_token=<access_token>"
},
"limits": {
"time": 1
},
"judge": {
"id": 1,
"name": "Ignores extra whitespaces",
"uri": "https://<endpoint>/api/v3/judges/1?access_token=<access_token>"
}
}
Updates the problem test case
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
number |
path | integer | test case number |
input | form | string | input data |
output | form | string | output data |
timeLimit | form | float | time limit [seconds] |
judgeId | form | integer | judge id |
active | form | boolean | whether test case should be active |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
404 | 1101 | testcase not found |
400 | 200302 | access to judge denied |
400 | 200301 | judge not found |
400 | 202205 | judge type is incorrect |
400 | 201905 | invalid format of time limit |
400 | 1103 | update failed |
Examples
$ cat request.json
{
"input": "updated content",
"timeLimit": 9,
"output": "updated content"
}
$ curl -X PUT \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/problems/:id/testcases/:number?access_token=<access_token>'
<?php
/**
* Example presents error handling for updateProblemTestcase() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 42;
$testcaseNumber = 0;
$newJudge = 2;
try {
$response = $client->updateProblemTestcase($problemId, $testcaseNumber, null, null, null, $newJudge);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for problems.updateTestcase() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
testcaseNumber = 0
newJudgeId = 1
try:
response = client.problems.updateTestcase(problemId, testcaseNumber, None, None, None, newJudgeId)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Non existing resource, error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
var testcaseNumber = 0;
var testcaseData = {
input: 'New input'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '/testcases/' + testcaseNumber + '?access_token=' + accessToken,
method: 'PUT',
form: testcaseData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log('Testcase updated');
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{}
Retrieves Problem test case file
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problemId parameter |
number |
path | integer | test case number |
filename |
path | string |
name of the file enum: input, output |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | problem not found |
404 | 1101 | testcase not found |
404 | 1201 | file not found |
400 | 1203 | reading file failed |
Examples
curl -X GET 'https://<endpoint>/api/v4/problems/:id/testcases/:number/:stream?access_token=<access_token>'
<?php
/**
* Example presents error handling for getProblemTestcaseFile() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$problemId = 42;
$testcaseNumber = 0;
$nonexistingFile = 'nonexistingFile';
try {
$response = $client->getProblemTestcaseFile($problemId, $testcaseNumber, $nonexistingFile);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the problem is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for problems.getTestcaseFile() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
problemId = 42
testcaseNumber = 0
file = 'input'
try:
response = client.problems.getTestcaseFile(problemId, testcaseNumber, file)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the problem is forbidden')
elif e.code == 404:
print('Non existing resource, error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var problemId = 42;
var testcaseNumber = 0;
var fileName = 'input'
// send request
request({
url: 'https://' + endpoint + '/api/v4/problems/' + problemId + '/testcases/' + testcaseNumber + '/' + fileName + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(response.body); // raw data from selected file
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
Raw data from selected file.
Creates a new judge
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
name | form | string | <empty> | judge name |
typeId | form | integer | 0 |
judge type enum:
|
compilerId |
form | integer | - | compiler id |
compilerVersionId | form | integer | - | compiler version identifier |
source |
form | string | - | source code |
shared | form | boolean | false | whether judge should be shared |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
400 | 201304 | source not provided |
400 | 202205 | judge type is incorrect |
400 | 201104 | compiler not provided |
400 | 201101 | compiler not found |
400 | 201108 | compiler not available |
400 | 1003 | creation failed |
Success Response
Field | Type | Description |
---|---|---|
id | integer | id of created judge |
Examples
$ cat request.json
{
"compilerId": 11,
"source": "<source code>"
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/judges?access_token=<access_token>'
<?php
/**
* Example presents error handling for createJudge() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$source = '<source code>';
$compiler = 11; // C language
try {
$response = $client->createJudge($source, $compiler);
// response['id'] stores the ID of the created judge
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for judges.create() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
source = '<source code>'
compiler = 11 # C language
try:
response = client.judges.create(source, compiler)
# response['id'] stores the ID of the created judge
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var judgeData = {
compilerId: 11,
compilerVersionId: 1,
source: '<source_code>'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/judges?access_token=' + accessToken,
method: 'POST',
form: judgeData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // judge data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"id": 42
}
Returns the list of judges of the quantity given by limit parameter starting from the offset parameter.
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
limit | query | integer | 10 |
limit of judges to get max: 100 |
offset | query | integer | 0 | offset |
typeId | query | integer | 0 |
judge type enum:
|
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
400 | 202205 | judge type is incorrect |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | judge id |
items[].name | string | judge name |
items[].type | object | |
items[].type.id | integer |
judge type id
|
items[].type.name | string |
judge type name
|
items[].visibility | object | |
items[].visibility.public | boolean | indicates whether the judge is public |
items[].visibility.shared | boolean | indicates whether the judge is shared |
items[].uri | string | link to judge details |
paging | object | |
paging.limit | integer | items per page |
paging.offset | integer | first item |
paging.total | integer | total number of items |
paging.pages | integer | total number of pages for the current limit |
paging.prev | string | previous page, link or null if no page |
paging.next | string | next page, link or null if no page |
Examples
curl -X GET 'https://<endpoint>/api/v4/judges?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful getJudges() API method
*/
use SphereEngine\Api\ProblemsClientV4;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$response = $client->getJudges();
"""
Example presents usage of the successful judges.all() API method
"""
from sphere_engine import ProblemsClientV4
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
response = client.judges.all()
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/judges?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of judges in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"items": [
{
"id": 1,
"name": "Ignores extra whitespaces",
"type": {
"id": 0,
"name": "testcase"
},
"visibility": {
"public": true,
"shared": true
},
"uri": "https://<endpoint>/api/v4/judges/1?access_token=<access_token>"
},
{
"id": 2,
"name": "Ignores FP rounding up to 10^-2",
"type": {
"id": 0,
"name": "testcase"
},
"visibility": {
"public": true,
"shared": false
},
"uri": "https://<endpoint>/api/v4/judges/2?access_token=<access_token>"
}
],
"paging": {
"limit": 2,
"offset": 4,
"total": 9,
"pages": 5,
"prev": "https://<endpoint>/api/v4/judges?offset=2&access_token=<access_token>",
"next": "https://<endpoint>/api/v4/judges?offset=6&access_token=<access_token>"
}
}
Retrieves an existing judge
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | judge id |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access to judge denied |
404 | 1001 | judge not found |
Success Response
Field | Type | Description |
---|---|---|
id | integer | judge id |
name | string | judge name |
type | object | |
type.id | integer |
judge type id
|
type.name | string |
judge type name
|
compiler | object | |
compiler.id | integer | compiler id |
compiler.name | string | compiler name |
compiler.version | object | compiler version |
compiler.version.id | integer | compiler version id |
compiler.version.name | string | compiler version name |
source | object | source code |
source.size | integer | source code length [bytes] |
source.uri | string | link to the file with source code |
visibility | object | |
visibility.public | boolean | indicates whether the judge is public |
visibility.shared | boolean | indicates whether the judge is shared |
Examples
curl -X GET 'https://<endpoint>/api/v4/judges/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for getJudge() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$judgeId = 1;
try {
$response = $client->getJudge($judgeId);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 404) {
echo 'Judge does not exist';
} elseif ($e->getCode() == 403) {
echo 'Access to the judge is forbidden';
}
}
"""
Example presents error handling for judges.get() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.judges.get(1)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 404:
print('Judge does not exist')
elif e.code == 403:
print('Access to the judge is forbidden')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var judgeId = 1;
// send request
request({
url: 'https://' + endpoint + '/api/v4/judges/' + judgeId + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // judge data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
if (response.statusCode === 403) {
console.log('Access denied');
}
if (response.statusCode === 404) {
console.log('Judge not found');
}
}
}
});
Response example
{
"id": 1,
"name": "Ignores extra whitespaces",
"type": {
"id": 0,
"name": "testcase"
},
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 5.1"
}
},
"source": {
"size": 160,
"uri": "https://<endpoint>/api/v4/judges/1/source?access_token=<access_token>"
},
"visibility": {
"public": true,
"shared": true
}
}
Updates an existing judge
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | judge id |
name | form | string | judge name |
compilerId | form | integer | compiler id |
compilerVersionId | form | integer | compiler version identifier |
source | form | string | source code |
shared | form | boolean | whether judge should be shared |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access to judge denied |
404 | 1001 | judge not found |
400 | 201101 | compiler not found |
400 | 201108 | compiler not available |
400 | 1003 | update failed |
Examples
$ cat request.json
{
"name": "<new_name>"
}
$ curl -X PUT -H 'Content-Type: application/json' -d "`cat request.json`" 'https://<endpoint>/api/v4/judges/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for updateJudge() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
$source = '<source code>';
$compiler = 11; // C language
try {
$response = $client->updateJudge(1, $source, $compiler);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the judge is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Judge does not exist';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for judges.update() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
source = '<source code>'
compiler = 11 # C language
try:
response = client.judges.update(1, source, compiler)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the judge is forbidden')
elif e.code == 404:
print('Judge does not exist')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var judgeId = 1;
var judgeData = {
compilerId: 11,
compilerVersionId: 1,
source: '<source_code>'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/judges/' + judgeId + '?access_token=' + accessToken,
method: 'PUT',
form: judgeData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log('Judge updated');
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
console.log('Judge does not exist');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{}
Retrieves an existing judge
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | judge id |
filename |
path | string | filename |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access to judge denied |
404 | 1001 | judge not found |
404 | 1101 | file not found |
400 | 1103 | reading file failed |
Examples
curl -X GET 'https://<endpoint>/api/v4/judges/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for getJudgeFile() API method
*/
use SphereEngine\Api\ProblemsClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new ProblemsClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getJudgeFile(1001, 'source');
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the judge is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for judges.getJudgeFile() API method
"""
from sphere_engine import ProblemsClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
try:
response = client.judges.getJudgeFile(1001, 'source')
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the judge is forbidden')
elif e.code == 404:
print('Non existing resource, error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var judgeId = 1;
var stream = 'source'
// send request
request({
url: 'https://' + endpoint + '/api/v4/judges/' + judgeId + '/' + stream + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(response.body); // raw data from selected stream
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
Raw data from selected stream.
Creates a new widget.
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
name |
form | string | - | widget name |
problem_id |
form | integer | - |
problem id Note on the backward compatibility: it is also allowed to use "the code of the problem" as the value of the problem_id parameter |
default_language |
form | integer | - | compiler id |
grade_mode | form | string | auto | grade mode (manual, auto) |
languages | form | array | - | list of languages |
date_from | form | string | - | start date and time for solution submissions [yyyy-mm-dd hh:mm:ss TZD] |
date_to | form | string | - | end date and time for solution submissions [yyyy-mm-dd hh:mm:ss TZD] |
source_code_template | form | string | - | Source code template |
max_submissions | form | integer | - | maximum number of submissions per user |
template_for_lp | form | string | - | template for Standalone page |
session_duration | form | integer | - | session duration |
show_ranking | form | boolean | true | show ranking |
show_user_data_on_ranking | form | boolean | false | display user data (i.e. name and/or login) publicly in the ranking |
show_welcome_form | form | boolean | false | show welcome form with *name* and *email* fields before allowing the user to enter the challenge |
secure_by_oauth | form | boolean | false | secure this widget by requiring the signature in the HTTP request |
display_test_cases_results | form | boolean | false | enable the user to see the results for each test case individually |
display_output | form | boolean | false | allow the user to view output data and error messages produced by the submission |
hide_submission_results | form | boolean | false | hide all details of the submission execution, including the final result, score, execution time, memory usage |
default_tab | form | string | problem |
default tab available tabs: problem, solve, history, ranking |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
403 | 3 | please contact with administrator to use widgets API |
400 | 2012004 | widget name not provided |
400 | 2012009 | empty widget name |
400 | 2012005 | widget name type is incorrect |
400 | 2012006 | widget name is too long |
400 | 200204 | problem not provided |
400 | 200201 | problem not found |
400 | 200202 | access to problem denied |
400 | 2012409 | empty default programming language |
400 | 2012405 | default language type is incorrect |
400 | 2012408 | default language not available |
400 | 1003 | creation failed |
400 | - | error occurred - please check your parameters; more info available in response |
Success Response
Field | Type | Description |
---|---|---|
hash | string | hash of created widget |
shared_secret | string | shared secret of created widget |
Examples
$ cat request.json
{
"name": "Example widget",
"problem_id": 42,
"default_language": 1
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
'https://<endpoint>/api/v4/widgets?access_token=<access_token>'
"""
Example presents usage of the successful widgets.create() API method
"""
from sphere_engine import ProblemsClientV4
import datetime
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = ProblemsClientV4(accessToken, endpoint)
# API usage
name = 'Example widget'
problem_id = 42
compiler = 11 # C language
# date_from = datetime.datetime.utcnow() + datetime.timedelta(hours=1)
response = client.widgets.create(name, problem_id, compiler)
# response['hash'] stores the hash of the created widget
Response example
{
"hash": "KhtGONpBh6",
"shared_secret": "75a6e7846349989b960b83d76eeb3934"
}