- Sphere Engine overview
- Compilers
- Overview
- API
- Widgets
- Resources
- Problems
- Overview
- API
- Widgets
- Handbook
- Resources
- Containers
- Overview
- Glossary
- API
- Workspaces
- Handbook
- Resources
- RESOURCES
- Programming languages
- Modules comparison
- Webhooks
- Infrastructure management
- API changelog
- FAQ
The Sphere Engine Containers API offers the ability to manage and execute complex multi-component programming projects. It provides integration methods for you to build platforms for teaching, verifying, and practicing programming skills, particularly in the areas of recruitment, education, e-learning, onboarding, and training.
Scope of functionality
With Sphere Engine Containers API, you can:
- send the end-user’s source code to be executed against a project,
- receive execution results,
- manage workspace sessions.
Web service
All URLs referring to the Sphere Engine Containers API have a common base called an endpoint:
https://<customer_id>.containers.sphere-engine.com/api/v<version>/
The Sphere Engine Containers API uses access tokens for authorization. The access tokens should be delivered in the
access_token
parameter for every call of an API method. The access tokens can be managed in the
API Tokens section of the Sphere Engine Client Panel.
For example, the test method available at a relative address /test
would be executed using the following URL:
https://<customer_id>.containers.sphere-engine.com/api/v1/test?access_token=abcdefgh
. In this example we used
customerId=12345678
, version=1
, and access_token=abcdefgh
.
The Sphere Engine Containers API is compliant with the REST architecture (learn more). This means:
- every API call is an
HTTP
request, - the
HTTP
request method specifies the purpose of the API call:GET
- retrieving data,POST
- creating a new resource,PUT
- updating an existing resource,DELETE
- deleting a resource,
- the
HTTP
response code informs about the outcome of the API method:- code beginning with 2 (e.g.,
200
,201
) indicates success, - code starting with 4 (e.g.,
404
) indicates an unsuccessful execution (e.g., a non-existent resource), - code beginning with 5 (e.g.,
500
) indicates a connection or server error.
- code beginning with 2 (e.g.,
Requests
The Sphere Engine API is a RESTful API, so the data traveling to and from the API is transferred over the HTTP
protocol. It means that any client library that supports HTTP
protocol can be used to integrate with Sphere Engine.
Most modern technologies offer a wide range of libraries and tools dedicated to RESTful APIs and the HTTP
protocol.
For GET
and DELETE
requests, you should provide the parameters in a query, i.e., as a part of an URL. For example:
https://<customer_id>.containers.sphere-engine.com/api/v1/submissions/42?access_token=<access_token>¶m=value
.
Both POST
and PUT
requests allow for parameters to be sent in three formats:
- JSON (i.e., Content-Type: application/json),
- FORM (i.e., Content-Type: application/x-www-form-urlencoded),
- MULTIPART (i.e., Content-Type: multipart/form-data).
Read on for a detailed description of all our API methods and their parameters.
Response format
The API response is a regular HTTP
response. It consists of an HTTP
response code and the message body.
The REST architecture uses HTTP
response codes to summarize the execution of the API methods. The table below lists
the response codes together with their interpretation:
Response code | Interpretation |
---|---|
200 |
success: GET , PUT and DELETE methods |
201 |
success: POST methods |
400 |
incorrect request (e.g., incorrect parameter format) |
401 |
authentication failed (i.e., missing or incorrect access token) |
403 |
access denied |
404 |
the requested resource doesn't exist |
5xx |
a family of server-side and connection-related errors |
The API responses are always returned in the JSON
format (i.e., Content-type: application/json).
In case of an execution error (i.e., 4xx
response code), the response structure is as follows:
{
"error_code": xxx,
"message": "message describing the error"
}
The error_code
field contains an integer that identifies the error and allows for its straightforward interpretation.
The API Reference covers a list of errors along with their corresponding
numerical values appearing in the error_code
field.
Note: The HTTP
response code and the error code (i.e., the error_code
field) are two different response
components.
Submission and a project
Let's see what submission and a project are:
- A source code submitted to Sphere Engine is called a submission,
- In the Sphere Engine Containers module, submissions are always associated with a project,
- A project is a docker container with custom files and libraries defined by the content manager,
- For each submission, the end-user's source code is incorporated into a project and then executed as a whole.
Submission status
In the response of an API method for retrieving submission data (i.e., GET /submissions/:id
) there are two fields
that define the current status of a submission.
Using the first field called executing
, you can quickly check whether Sphere Engine is still processing the
submission or has already finished doing so:
executing = true
- Sphere Engine is still processing the submission; at this point, the data generated by the submission is not yet available,executing = false
- at this point, the submission has been fully executed; all the information (e.g., execution time, memory consumption, data streams) has been established and is ready to be fetched.
The second integer field, called status
, accurately determines the current status of the submission. Below is a
complete list of submission states:
List of transitional states (i.e., executing = true
)
Status | Name | Description |
---|---|---|
0 | waiting | the submission is waiting in the queue |
1 | preparation | the environment for the execution is being prepared |
3 | execution | the submission is being executed |
List of final states (i.e., executing = false
)
Status | Name | Description |
---|---|---|
11 | build error | the submission's execution failed during the build stage |
12 | runtime error | an error occurred during the submission's execution (e.g., division by zero) |
13 | time limit exceeded | the submission exceeded the time limit |
14 | fail | the submission's execution was considered unsuccessful during evaluation stage |
15 | ok | the submission has been executed successfully |
20 | internal error | an unexpected error occurred on the Sphere Engine's side; try resubmitting the submission; please contact us if the error persists |
22 | project error | the submission's execution failed due to an error in the project configuration; refer to debug_log for more information |
Workspace state
The response of the API method for retrieving the workspace data (i.e., GET /workspaces/:code
for a specific
workspace and GET /workspaces
for a list of them) contains a field state.code
defining the current state
of the workspace. The following table summarizes the meaning of state.code
values:
List of workspace states:
Code | Name | Description |
---|---|---|
1 | starting | workspace is starting |
2 | running | workspace is running |
3 | stopped | workspace is stopped |
4 | removed | workspace was removed |
5 | error | workspace produced an internal error |
The following diagram presents the life-cycle of the workspace assuming using stop/restore functionality. It shows the different states and the transitions between them.
Waiting for the submission to be executed
When you create a submission, Sphere Engine needs a certain amount of time to execute it. Follow the steps below to get the results of the submission execution:
- Create a submission using the
POST /submissions
method, - Wait 5 seconds,
- Retrieve submission data using the
GET /submissions/:id
method, - [optional] Present the current status of the submission to the user,
- If
executing = true
, go back to step /2/, - [optional] Present the execution results to the user.
The diagram below presents the concept behind these steps. The timeline shown in the diagram is an example, and you should adjust it based on your needs.
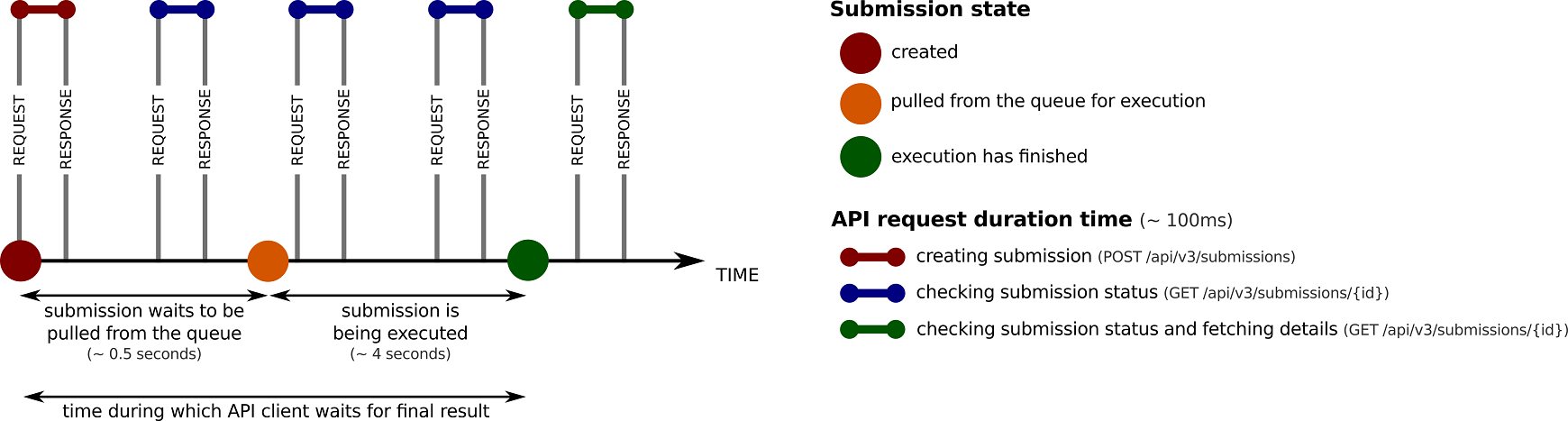
Important: Intensive server polling (i.e., no time interval between API requests) while waiting for a submission to be processed can result in a response from the Sphere Engine's security system. A high volume of API requests can be interpreted as a DoS/DDoS attack. In such cases, Sphere Engine servers may refuse further access to the service.
API methods
The technical reference for the API is available in a separate document: