- Sphere Engine overview
- Compilers
- Overview
- API
- Widgets
- Resources
- Problems
- Overview
- API
- Widgets
- Handbook
- Resources
- Containers
- Overview
- Glossary
- API
- Workspaces
- Handbook
- Resources
- RESOURCES
- Programming languages
- Modules comparison
- Webhooks
- Infrastructure management
- API changelog
- FAQ
Important: If you are just starting with Sphere Engine Compilers API, please use its latest version.
Introduction
This document describes Sphere Engine Compilers web service. It is explained here how the methods should be used and how to interpret data returned by them. All URLs referenced in the documentation have the base https://<customer_id>.compilers.sphere-engine.com/api/v3/
.
Functionality
Sphere Engine Compilers API is designed for:
- uploading a source code of the program,
- executing the program using various compilers,
- executing programs with input data,
- fetching results of the execution, including:
- generated output,
- standard error,
- compilation information,
- execution time,
- consumed memory.
The web service
All API URLs listed in this document are relative to the https://<customer_id>.compilers.sphere-engine.com/api/v3/
link called an endpoint. For example, the /test API call is reachable at https://<customer_id>.compilers.sphere-engine.com/api/v/test?access_token=<access_token>
.
The Sphere Engine Compilers API is a RESTful API. This implies the following:
- API calls should be made with
HTTP
requests (GET, POST, etc.), - successes are represented by the 2xx codes,
- errors are represented by 4xx and 5xx codes,
- error code from 4xx group represents client errors,
- error code from 5xx group represents connection or server error.
Requests
Request data is passed to the API by posting parameters to the API endpoints. To communicate with API endpoint you can
use any HTTP
client/library. Each function takes at least one parameters - the access_token
. For every GET
and
DELETE
request parameters have to be submitted in the query (i.e., url
). For POST
and PUT
request you can send
parameters in the two possible ways:
- as a
FORM
(i.e., Content-Type: application/x-www-form-urlencoded), - as a
JSON
(i.e., Content-Type: application/json).
The documentation for each API call will contain more details on the parameters accepted by the call.
Responses
Each response has two components: HTTP
Status Code and JSON
-encoded message.
The RESTful API uses HTTP
response code to communicate the success or error. Here is the general list of possible codes for Sphere Engine Compilers API.
Value | Interpretation |
---|---|
200 | success |
201 | success (for creation methods) |
400 | bad request (invalid parameters) |
401 | unauthorized access (missing or incorrect access token) |
403 | forbidden access (referring to foreign or secured content) |
404 | non existing content (submission or compiler) |
5xx | server side problem |
Status and result
Variables status and result returned with submission details (i.e., using GET /submissions/:id
API method) require
further explanation.
Status specifies the stage of program's execution. Its values should be interpreted in the following way:
Value | Name | Description |
---|---|---|
< 0 | waiting for compilation | the submission awaits execution in the queue |
0 | finished | the program has finished |
1 | compilation | the program is being compiled |
3 | running | the program is being executed |
It's a common practice to implement the following scenario for waiting for execution: 1. submit source code, 1. wait 5 seconds, 1. retrieve submission details, 1. (optional) present current status to the user, 1. if the status <> 0 go back to step (2), 1. (optional) present all execution details to the user.
The following diagram presents the idea of this algorithm:
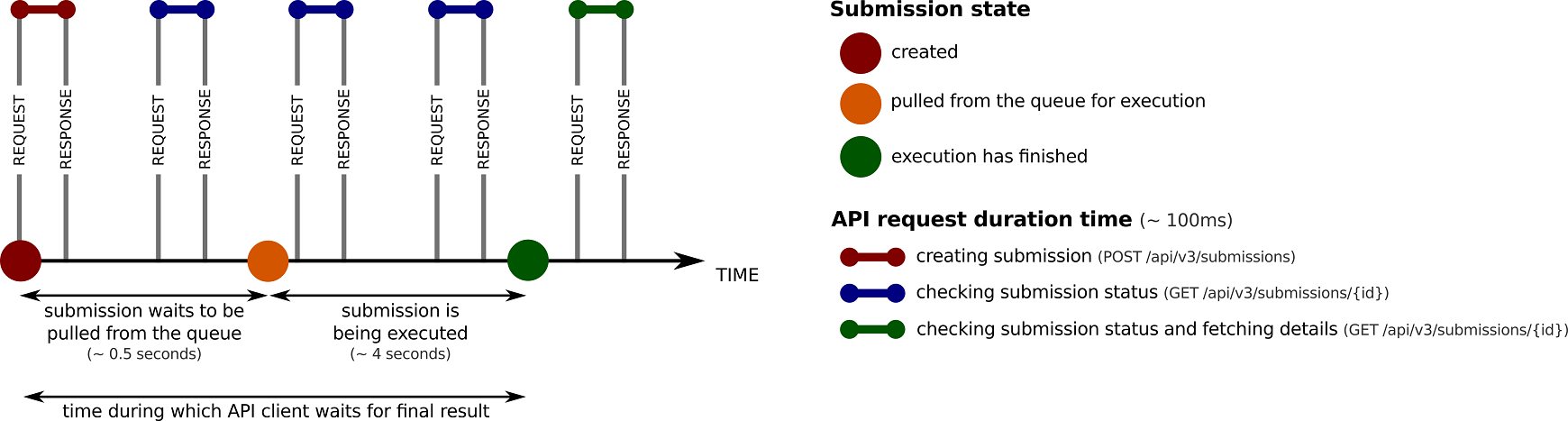
When the submission's status is 0 you can find out how the program has finished by checking the result variable.
Value | Name | Description |
---|---|---|
11 | compilation error | the program could not be executed due to compilation error |
12 | runtime error | the program finished because of the runtime error, for example: division by zero, array index out of bounds, uncaught exception |
13 | time limit exceeded | the program didn't stop before the time limit |
15 | success | everything went ok |
17 | memory limit exceeded | the program tried to use more memory than it is allowed to |
19 | illegal system call | the program tried to call illegal system function |
20 | internal error | some problem occurred on Sphere Engine; try to submit the program again and if that fails too, then please contact us |
Binary data
The output data produced by a program doesn't need to be a text file (i.e., human-readable string). If the output stream is a binary file then the response for the submission details API method call contains URL to the raw output stream.
If the output data is an image (png
, jpg
, gif
, bmp
) then this information is reflected in the API response
(see. the output_type
field). An extended discussion related to outputting graphical data is covered in the
separate section.
Client libraries
There are client libraries available for the following programming languages:
In addition, there are also examples of using the Sphere Engine API for several other technologies:
For each API
method below, there is an example of calling this method from the client library or using selected
technologies.
Backward compatibility
If you are a user of Ideone API please take into consideration that it is no longer under development process. We highly recommend migrating to the current version. However, the Ideone API is still available and introduction of the new version will not affect your services built with previous versions.
The WSDL endpoint address will be available the following address:
https://<customer_id>.compilers.sphere-engine.com/api/1/service.wsdl
We are going to maintenance this endpoint up to release of next version of our API. Since that time we stop maintaining Ideone API and the only supported API will be the new REST API.
API methods
For testing purposes. Returns the same data every time it is called (if wrong token is provided, then AUTH_ERROR error code is returned).
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
Code | Description |
---|---|
200 | success |
401 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
moreHelp | string | hints where to find more information |
pi | float | approximate value of π |
answerToLifeAndEverything | integer | as far as we know it's 42 |
oOok | boolean | boolean field |
Examples
curl -X GET 'https://<endpoint>/api/v3/test?access_token=<access_token>'
package compilers;
/**
* Example presents usage of the successful test() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class test
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
try {
JsonObject response = client.test();
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents usage of the successful test() API method
*/
use SphereEngine\Api\CompilersClientV3;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
try {
$response = $client->test();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful test() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
try:
response = client.test()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v3/test?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // test message in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class test {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v3/test?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v3/test?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"moreHelp": "For more help please log in to Sphere Engine and go to Compilers -> API Documentation.",
"pi": 3.14,
"answerToLifeAndEverything": 42,
"oOok": true
}
Returns a list of supported compilers.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
Code | Description |
---|---|
200 | success |
401 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | compiler id |
items[].name | string | compiler name |
items[].ver | string | compiler version |
items[].short | string | short name |
items[].ace | string | syntax highlighting identifier for Ace |
items[].geshi | string | syntax highlighting identifier for Geshi |
items[].pygments | string | syntax highlighting identifier for Pygments |
items[].highlights | string | syntax highlighting identifier for Highlights |
items[].rouge | string | syntax highlighting identifier for Rouge |
items[].codemirror | string | syntax highlighting identifier for CodeMirror |
items[].highlightjs | string | syntax highlighting identifier for highlight.js |
items[].prism | string | syntax highlighting identifier for Prism |
items[].monaco | string | syntax highlighting identifier for Monaco Editor |
items[].code_prettify | string | syntax highlighting identifier for Google Code Prettify |
Examples
curl -X GET 'https://<endpoint>/api/v3/compilers?access_token=<access_token>'
package compilers;
/**
* Example presents usage of the successful getCompilers() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class getCompilers
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
try {
JsonObject response = client.getCompilers();
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents usage of the successful getCompilers() API method
*/
use SphereEngine\Api\CompilersClientV3;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
try {
$response = $client->getCompilers();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful compilers() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
try:
response = client.compilers()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v3/compilers?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of compilers in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getCompilers {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v3/compilers?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v3/compilers?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"items": [
{
"id": 1,
"name": "C++",
"ver": "gcc 6.3",
"short": "CPP",
"ace": "c_cpp",
"geshi": "cpp",
"pygments": "cpp",
"highlights": "c",
"rouge": "cpp",
"codemirror": "clike",
"highlightjs": "cpp",
"prism": "cpp",
"monaco": "cpp",
"code_prettify": "cpp"
},
{
"id": 116,
"name": "Python 3",
"ver": "3.5",
"short": "PYTHON3",
"ace": "python",
"geshi": "python",
"pygments": "python",
"highlights": "python",
"rouge": "python",
"codemirror": "python",
"highlightjs": "python",
"prism": "python",
"monaco": "python",
"code_prettify": "python"
}
]
}
Returns information about a submission.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | submission id |
withSource | formData | boolean | whether source code of the submission should be returned |
withInput | formData | boolean | whether input data of the submission should be returned |
withOutput | formData | boolean | whether output produced by the program should be returned |
withStderr | formData | boolean | whether stderr should be returned |
withCmpinfo | formData | boolean | whether compilation information should be returned |
Response HTTP Code
Code | Description |
---|---|
200 | success |
401 | unauthorized access |
403 | access denied |
404 | submission not found |
Success Response
Field | Type | Description |
---|---|---|
time | float | execution time in seconds |
memory | integer | memory consumed by the program in kilobytes |
date | string | creation date and time (on server); format: yyyy-mm-dd hh:mm:ss; eg. 2009-05-19 02:34:56 |
status | integer | current status; see section Status and result |
result | integer | current result; see section Status and result |
signal | integer | signal raised by the program |
compiler.id | integer | compiler id |
compiler.name | string | compiler name |
compiler.ver | string | compiler version |
source | string | source code of the submission; this value is returned if the withSource parameter is set to true |
input | string | input data of the submission; this value is returned if the withInput parameter is set to true |
output | string | output produced by the program or url to raw output stream if the output data is a binary file; this value is returned if the withOutput parameter is set to true |
output_type | string | content type of the output stream; text, image or binary; output stream is recognized as an image only for PNG, JPG, GIF and BMP formats; this value is returned if the withOutput parameter is set to true |
stderr | string | stderr produced by the program; this value is returned if the withStderr parameter is set to true |
cmpinfo | string | compilation information regarding the program; this value is returned if the withCmpinfo parameter is set to true |
Examples
curl -X GET 'https://<endpoint>/api/v3/submissions/:id?access_token=<access_token>'
package compilers.submissions;
/**
* Example presents usage of the successful getSubmission() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.NotFoundException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class getSubmission
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
try {
JsonObject response = client.getSubmission(2016);
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (NotFoundException e) {
System.out.println("Submission does not exist");
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents error handling for getSubmission() API method
*/
use SphereEngine\Api\CompilersClientV3;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
try {
$nonexisting_submission_id = 999999999;
$response = $client->getSubmission($nonexisting_submission_id);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 404) {
echo 'Submission does not exist';
}
}
"""
Example presents error handling for submissions.get() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
try:
nonexisting_submission_id = 999999999;
response = client.submissions.get(nonexisting_submission_id)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 404:
print('Submission does not exist')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 2016;
// send request
request({
url: 'https://' + endpoint + '/api/v3/submissions/' + submissionId + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
if (response.statusCode === 403) {
console.log('Access denied');
}
if (response.statusCode === 404) {
console.log('Submision not found');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
// define request parameters
int submissionId = 2016;
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v3/submissions/" + submissionId + "?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
} else if (statusCode == HttpStatusCode.Forbidden) {
Console.WriteLine("Access denied");
} else if (statusCode == HttpStatusCode.NotFound) {
Console.WriteLine("Submission not found");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_id = "2016"
# send request
uri = URI.parse("https://" + endpoint + "/api/v3/submissions/" + submission_id + "?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
when Net::HTTPForbidden
puts "Access denied"
when Net::HTTPNotFound
puts "Submission not found"
end
rescue => e
puts "Connection error"
end
Response example
{
"time": 0.4,
"memory": 2048,
"date": "2017-02-24 14:24:21",
"status": 0,
"result": 0,
"signal": 0,
"compiler": {
"id": 1,
"name": "C++",
"ver": "gcc 6.3"
}
}
Returns raw stream data.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | submission id |
stream |
path | integer | stream name (input, output, stderr, cmpinfo or source) |
Response HTTP Code
Code | Description |
---|---|
200 | success |
401 | unauthorized access |
403 | access denied |
404 | submission not found | stream not found |
Examples
curl -X GET 'https://<endpoint>/api/v3/submissions/:id/output?access_token=<access_token>'
package compilers.submissions;
/**
* Example presents usage of the successful getSubmissionStream() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.NotFoundException;
import com.SphereEngine.Api.Exception.BadRequestException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class getSubmissionStream
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
try {
String response = client.getSubmissionStream(2016, "output");
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (NotFoundException e) {
// aggregates two possible reasons of 404 error
// non existing submission or stream
System.out.println("Non existing resource (submission, stream), details available in the message: " + e.getMessage());
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents error handling for getSubmissionStream() API method
*/
use SphereEngine\Api\CompilersClientV3;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
try {
$nonexisting_submission_id = 999999999;
$response = $client->getSubmissionStream($nonexisting_submission_id, 'output');
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 404) {
// aggregates two possible reasons of 404 error
// non existing submission or stream
echo 'Non existing resource (submission, stream), details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.getStream() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
try:
response = client.submissions.getStream(2016, 'nonexistingstream')
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 404:
# aggregates two possible reasons of 404 error
# non existing submission or stream
print('Non existing resource (submission, stream), details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 2016;
// send request
request({
url: 'https://' + endpoint + '/api/v3/submissions/' + submissionId + '/input?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(response.body); // raw data from selected stream
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
if (response.statusCode === 403) {
console.log('Access denied');
}
if (response.statusCode === 404) {
console.log('Submision or stream not found');
}
}
}
});
Response example
Raw data from selected stream.
Creates a new submission. Note that every usage of this method decreases submissions pool for your account.
If you want to create a submission with many files see: multi-file mode.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
source | formData | string | source code, default: empty (see also: multi-file mode) |
compilerId | formData | integer | compiler identifier, default: 1 (C++) |
input | formData | string | data that will be provided to the program as stdin stream, default: empty |
Response HTTP Code
Code | Description |
---|---|
201 | success |
401 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
id | integer | id of created submission |
Examples
$ cat request.json
{
"language": 11,
"sourceCode": "<source code>"
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
"https://<endpoint>/api/v3/submissions?access_token=<access_token>"
package compilers.submissions;
/**
* Example presents usage of the successful createSubmission() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class createSubmission
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
String source = "<source code>";
Integer compiler = 11; // C language
String input = "2016";
try {
JsonObject response = client.createSubmission(source, compiler, input);
// response.get("id") stores the ID of the created submission
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents error handling for createSubmission() API method
*/
use SphereEngine\Api\CompilersClientV3;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
$source = '<source code>';
$compiler = 11; // C language
$input = '2016';
try {
$response = $client->createSubmission($source, $compiler, $input);
// response['id'] stores the ID of the created submission
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents error handling for submissions.create() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
source = '<source code>'
compiler = 11 # C language
input = '2016'
try:
response = client.submissions.create(source, compiler, input)
# response['id'] stores the ID of the created submission
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionData = {
language: 11,
sourceCode: '<source_code>'
};
// send request
request({
url: 'https://' + endpoint + '/api/v3/submissions?access_token=' + accessToken,
method: 'POST',
form: submissionData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class createSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient ();
// define request parameters
NameValueCollection formData = new NameValueCollection ();
formData.Add("source", "<source_code>");
formData.Add("compilerId", "11");
// send request
byte[] responseBytes = client.UploadValues("https://" + endpoint + "/api/v3/submissions?access_token=" + accessToken, "POST", formData);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse)response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_data = {
"language" => "11",
"sourceCode" => '<source_code>'
};
# send request
uri = URI.parse("https://" + endpoint + "/api/v3/submissions?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri);
request.set_form_data(submission_data)
begin
response = http.request(request)
# process response
case response
when Net::HTTPCreated
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"id": 42
}
Returns information about submissions. Results are sorted ascending by id.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
ids |
query | string | ids of submissions separated by comma, maximum 20 ids |
Response HTTP Code
Code | Description |
---|---|
200 | success |
401 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | submission id |
items[].time | float | execution time in seconds |
items[].memory | integer | memory consumed by the program in kilobytes |
items[].date | string | creation date and time (on server); format: yyyy-mm-dd hh:mm:ss; eg. 2009-05-19 02:34:56 |
items[].status | integer | current status; see section Status and result |
items[].result | integer | current result; see section Status and result |
items[].signal | integer | signal raised by the program |
items[].compiler.id | integer | compiler id |
items[].compiler.name | string | compiler name |
items[].compiler.ver | string | compiler version |
Examples
curl -X GET 'https://<endpoint>/api/v3/submissions?ids=:ids&access_token=<access_token>'
package compilers.submissions;
/**
* Example presents usage of the successful getSubmissions() API method
*/
import com.SphereEngine.Api.CompilersClientV3;
import com.SphereEngine.Api.Exception.NotAuthorizedException;
import com.SphereEngine.Api.Exception.ClientException;
import com.SphereEngine.Api.Exception.ConnectionException;
import com.google.gson.JsonObject;
public class getSubmissions
{
public static void main(String[] args)
{
CompilersClientV3 client = new CompilersClientV3(
"<access_token>",
"<endpoint>");
try {
Integer[] ids = new Integer[2];
ids[0] = 2017;
ids[1] = 2018;
JsonObject response = client.getSubmissions(ids);
} catch (NotAuthorizedException e) {
System.out.println("Invalid access token");
} catch (ClientException e) {
System.out.println(e.getMessage());
} catch (ConnectionException e) {
System.out.println(e.getMessage());
}
}
}
<?php
/**
* Example presents error handling for getSubmissions() API method
*/
use SphereEngine\Api\CompilersClientV3;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV3($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmissions(array(2017, 2018));
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents error handling for submissions.getMulti() API method
"""
from sphere_engine import CompilersClientV3
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV3(accessToken, endpoint)
# API usage
try:
response = client.submissions.getMulti([2017, 2018])
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionsIds = [2016, 2017];
// send request
request({
url: 'https://' + endpoint + '/api/v3/submissions?ids=' + submissionsIds.join() + '&access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of submissions in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
Response example
{
"items": [
{
"id": 1,
"time": 0.4,
"memory": 2048,
"date": "2017-02-24 14:24:21",
"status": 0,
"result": 0,
"signal":0,
"compiler": {
"id": 1,
"name": "C++",
"ver": "gcc 6.3"
}
}
]
}