- Sphere Engine overview
- Compilers
- Overview
- API
- Widgets
- Resources
- Problems
- Overview
- API
- Widgets
- Handbook
- Resources
- Containers
- Overview
- Glossary
- API
- Workspaces
- Handbook
- Resources
- RESOURCES
- Programming languages
- Modules comparison
- Webhooks
- Infrastructure management
- API changelog
- FAQ
Sphere Engine Compilers API offers the ability to execute programs in dozens of programming languages, including C++, C#, Go, Haskell, Java, Kotlin, Node.js, PHP, Python, Ruby, Scala, or Swift among others (full list of supported programming languages).
When running a program, you can specify:
- the source code of the program,
- the programming language,
- input data (optional).
Data available after the program is executed includes:
- execution time,
- memory consumption,
- exit signal,
- data streams containing:
- generated output data,
- runtime errors,
- warnings and compilation errors.
For a full list of retrievable data please refer to the Sphere Engine Compilers API Docs.
Important: To successfully use Sphere Engine Compilers API you need to have a working Sphere Engine account with a dedicated API endpoint and API token. Register for a Sphere Engine account by filling in the sign-up form.
Web service
All URLs referring to the Sphere Engine Compilers API have a common base: https://<customer_id>.compilers.sphere-engine.com/api/v4/
called an
endpoint. We authorize users using an access token which should be given as a parameter named access_token for
every use of the API method. The management of access tokens is available in Sphere Engine client panel
API Tokens section.
For example, the test method available at a relative address /test
should be executed using the following address
along with the access token: https://<customer_id>.compilers.sphere-engine.com/api/v4/test?access_token=<access_token>
.
The Sphere Engine Compilers API is compliant with the REST architecture. This results in the following characteristics of the service:
- every API use is an
HTTP
request, - the
HTTP
request method specifies the type of the API method:GET
– retrieving data,POST
– creating a new resource,PUT
– updating the data of the resource,DELETE
– deleting the resource,
- The
HTTP
response code informs about the execution of the API method:- codes beginning with 2 (e.g.,
200
,201
) inform about success, - codes starting with 4 (e.g.,
404
) inform about the unsuccessful execution of the method (e.g., referring to a non-existent resource), - codes beginning with 5 (e.g.,
500
) inform about connection or server errors.
- codes beginning with 2 (e.g.,
Requests
Transfer of data to the API is done using HTTP
parameters. Therefore, any client library that supports the HTTP
protocol can be used to integrate with the Sphere Engine service. Most modern application production technologies offer
a wide range of solutions enabling HTTP
communication. You can also find solutions dedicated to supporting RESTful
API services.
For GET
and DELETE
requests, parameters should be included in the query, i.e., as part of the URL. For example:
https://<customer_id>.compilers.sphere-engine.com/api/v4/submissions?access_token=<access_token>&ids=40,41,42
.
POST
and PUT
requests allow for sending parameters in three formats:
- JSON (i.e., Content-Type: application/json),
- FORM (i.e., Content-Type: application/x-www-form-urlencoded),
- MULTIPART (i.e., Content-Type: multipart/form-data).
Read on for a detailed description of parameters available for every API method.
Response format
The API response is an HTTP
response. It consists of HTTP
status and message body.
The REST
architecture uses HTTP
response codes to summarize the execution of the API method. The table below lists
the response codes together with their interpretation.
Response code | Interpretation |
---|---|
200 | success in GET , PUT and DELETE methods |
201 | success in POST methods |
400 | incorrect request (e.g., incorrect parameter format) |
401 | authentication failed (i.e., missing or incorrect access token) |
403 | lack of authorization to access the resource |
404 | the requested resource does not exist |
5xx | a family of errors occurring server-side or connection-side |
The response is always returned in the JSON
format (i.e., Content-type: application/json). In case of an API method
execution error (i.e., 4**
response code), the structure of the response is as follows:
{
"error_code": xxx,
"message": "message describing the error"
}
The error_code
field contains an integer identifying the error and allowing for its easy interpretation. The detailed
description of the API methods contains a list of errors along with their corresponding numerical values which appear in
the error_code
field.
Note: The HTTP
response code and the error code (i.e., the error_code
field) are two different response
components.
Submission status
The programs sent to be executed using the Sphere Engine system are called submissions. A submission consists of the source code of the program, the programming language and (optionally) the input data to be processed by the program.
The response of the API method for retrieving the data of submission (i.e., GET /submissions/:id
) contains two fields
defining the current status of the submission.
The binary executing
field helps you quickly verify if the submission is being processed by the system. This is
determined by the values:
true
- the submission is still being processed by the system. At this point, the data generated by the program is not yet available,false
- at this point, the submission has already left the part of the Sphere Engine system responsible for the execution of programs. All information (e.g., execution time, memory consumption, data streams) has been established and is available.
The integer field called status
precisely determines the status of the submission. Values should be interpreted by
the following tables:
List of transient states (i.e., executing = true
)
Status | Name | Description |
---|---|---|
0 | waiting | submission is waiting in the queue |
1,2 | compilation | program is being compiled (for compiled languages) |
3 | execution | program is being executed |
List of final states (i.e., executing = false
)
Status | Name | Description |
---|---|---|
11 | compilation error | the program could not be executed due to a compilation error |
12 | runtime error | an error occurred while the program was running (e.g., division by zero) |
13 | time limit exceeded | the program exceeded the time limit |
15 | success | the program was executed correctly |
17 | memory limit exceeded | the program exceeded the memory limit |
19 | illegal system call | the program tried to call illegal system function |
20 | internal error | an unexpected error occurred on the Sphere Engine side try making the submission again and if this occurs again, please contact us |
Waiting for the submission to be executed
Note: To get faster results and bypass polling we recommend using the webhook integration.
After creating a new submission in the system, some time is required for it to be executed. A recommended practice while waiting for the submission to finish processing is to implement the following scenario:
- Create a submission using the
POST /submissions
method. - Wait 2 seconds.
- Retrieve submission data using the
GET /submissions/:id
method. - [optional] Present the current status of the submission to the user.
- If executing = true, go back to step /2/.
- [optional] Present the data of the submission to the user.
The diagram below presents the concept of this scenario. The duration shown in the diagram is hypothetical:
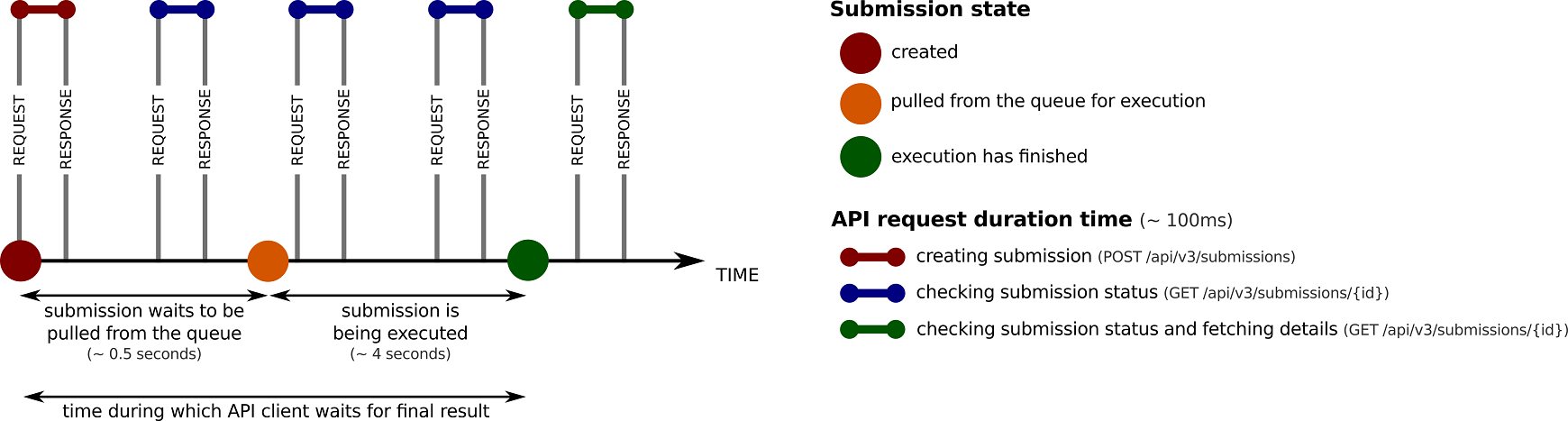
Important: Intensive server polling (i.e., no time interval between requests) while waiting for a submission to be processed can lead to a reaction from the Sphere Engine API security system. High intensity of API requests can be interpreted as a DoS/DDoS attack. In this case, Sphere Engine servers may refuse further access to the service.
Binary data
The output data produced by a program doesn't need to be a text file (i.e., human-readable string). If the output stream is a binary file then the response for the submission details API method call contains URL to the raw output stream.
If the output data is an image (png
, jpg
, gif
, bmp
) then this information is reflected in the API response
(see. the output_type
field). An extended discussion related to outputting graphical data is covered in the
separate section.
Client libraries
There are client libraries available for the following programming languages:
In addition, there are also examples of using the Sphere Engine API for several other technologies:
For each API
method below, there is an example of calling this method from the client library or using selected
technologies.
Migration from previous versions
Due to extensive changes that were made when creating version 4 of the Sphere Engine API, we were unable to maintain compatibility with its previous version.
However, the migration process to the current version shouldn't be troublesome. From the user's perspective, most of the changes are related to the structure of the parameters of the API methods and the structure of the response.
Refer to the full list of changes for details.
API methods
Testing Compilers API. Every successful usage returns identical response.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
message | string | message that states that it's possible to use API |
Examples
curl -X GET 'https://<endpoint>/api/v4/test?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful test() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->test();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful test() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
try:
response = client.test()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/test?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // test message in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class test {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/test?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/test?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"message": "You can use Sphere Engine Compilers API."
}
Returns a list of supported compilers.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | compiler id |
items[].name | string | compiler name |
items[].short | string | short name |
items[].versions[].id | integer | compiler version id |
items[].versions[].name | string | compiler version name |
items[].highlighters | object | syntax highlighters |
items[].highlighters.ace | string | syntax highlighting identifier for Ace |
items[].highlighters.geshi | string | syntax highlighting identifier for Geshi |
items[].highlighters.pygments | string | syntax highlighting identifier for Pygments |
items[].highlighters.highlights | string | syntax highlighting identifier for Highlights |
items[].highlighters.rouge | string | syntax highlighting identifier for Rouge |
items[].highlighters.codemirror | string | syntax highlighting identifier for CodeMirror |
items[].highlighters.highlightjs | string | syntax highlighting identifier for highlight.js |
items[].highlighters.prism | string | syntax highlighting identifier for Prism |
items[].highlighters.monaco | string | syntax highlighting identifier for Monaco Editor |
items[].highlighters.code_prettify | string | syntax highlighting identifier for Google Code Prettify |
Examples
curl -X GET 'https://<endpoint>/api/v4/compilers?access_token=<access_token>'
<?php
/**
* Example presents usage of the successful getCompilers() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getCompilers();
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
}
}
"""
Example presents usage of the successful compilers() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
try:
response = client.compilers()
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// send request
request({
url: 'https://' + endpoint + '/api/v4/compilers?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of compilers in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getCompilers {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/compilers?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/compilers?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
end
rescue => e
puts "Connection error"
end
Response example
{
"items": [
{
"id": 1,
"name": "C++",
"short": "CPP",
"versions": [
{
"id": 1,
"name": "gcc 5.1"
},
{
"id": 2,
"name": "gcc 6.3"
}
],
"highlighters": {
"ace": "c_cpp",
"geshi": "cpp",
"pygments": "cpp",
"highlights": "c",
"rouge": "cpp",
"codemirror": "clike",
"highlightjs": "cpp",
"prism": "cpp",
"monaco": "cpp",
"code_prettify": "cpp"
}
},
{
"id": 116,
"name": "Python 3",
"short": "PYTHON3",
"versions": [
{
"id": 1,
"name": "3.5"
}
],
"highlighters": {
"ace": "python",
"geshi": "python",
"pygments": "python",
"highlights": "python",
"rouge": "python",
"codemirror": "python",
"highlightjs": "python",
"prism": "python",
"monaco": "python",
"code_prettify": "python"
}
}
]
}
Creates a new submission. You can create a submission with many files using multi-file mode. Every usage of this method decreases submissions pool for your account.
Request parameters
Name | Located in | Type | Default value | Description |
---|---|---|---|---|
access_token |
query | string | - | access token |
source |
form | string | - | source code (see also: multi-file mode) |
compilerId |
form | integer | - | compiler identifier |
compilerVersionId | form | integer | 1 | compiler version identifier |
input | form | string | <empty> | data that will be provided to the program as input stream |
files | form | array | <no files> | set of files that form a project to be executed (see also: multi-file mode) |
timeLimit | form | integer | 5 | time limit [seconds] |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
201 | - | success |
401 | 1 | unauthorized access |
402 | 2 | inactive account or empty submissions poll |
400 | 201304 | source not provided |
400 | 201101 | compiler not found |
400 | 201201 | compiler version not found |
400 | 201107 | compiler not supported for multi-files submissions |
400 | 201104 | compiler not provided |
400 | 201306 | source size limit exceeded |
400 | 201406 | input size limit exceeded |
400 | 201906 | time limit out of range |
400 | 202006 | memory limit out of range |
400 | 1003 | creation failed |
Success Response
Field | Type | Description |
---|---|---|
id | integer | id of created submission |
Examples
$ cat request.json
{
"compilerId": 11,
"source": "<source code>"
}
$ curl -X POST \
-H 'Content-Type: application/json' \
-d "`cat request.json`" \
"https://<endpoint>/api/v4/submissions?access_token=<access_token>"
<?php
/**
* Example presents error handling for createSubmission() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
$source = '<source code>';
$compiler = 11; // C language
$input = '2017';
try {
$response = $client->createSubmission($source, $compiler, $input);
// response['id'] stores the ID of the created submission
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 402) {
echo 'Unable to create submission';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.create() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
source = '<source code>'
compiler = 11 # C language
input = '2017'
try:
response = client.submissions.create(source, compiler, input)
# response['id'] stores the ID of the created submission
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 402:
print('Unable to create submission')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionData = {
compilerId: 11,
source: '<source_code>'
};
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions?access_token=' + accessToken,
method: 'POST',
form: submissionData
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 201) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 402) {
console.log('Unable to create submission');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class createSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
try {
WebClient client = new WebClient ();
// define request parameters
NameValueCollection formData = new NameValueCollection ();
formData.Add("source", "<source_code>");
formData.Add("compilerId", "11");
// send request
byte[] responseBytes = client.UploadValues("https://" + endpoint + "/api/v4/submissions?access_token=" + accessToken, "POST", formData);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse)response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
} else if(statusCode == HttpStatusCode.PaymentRequired) {
Console.WriteLine("Unable to create submission");
} else if(statusCode == HttpStatusCode.BadRequest) {
StreamReader reader = new StreamReader(response.GetResponseStream(), Encoding.UTF8);
Console.WriteLine(reader.ReadToEnd());
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_data = {
"compilerId" => "11",
"source" => '<source_code>'
};
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/submissions?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
request = Net::HTTP::Post.new(uri.request_uri);
request.set_form_data(submission_data)
begin
response = http.request(request)
# process response
case response
when Net::HTTPCreated
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
when Net::HTTPPaymentRequired
puts "Unable to create submission"
when Net::HTTPBadRequest
body = JSON.parse(response.body)
puts "Error code: " + body["error_code"].to_s + ", details available in the message: " + body["message"].to_s
end
rescue => e
puts "Connection error"
end
Response example
{
"id": 42
}
Returns information about submissions. Results are sorted ascending by id.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
ids |
query | string |
comma separated list of submission identifiers maximum number of identifiers: 20 |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
400 | 205104 | identifiers are not provided |
400 | 205105 | invalid format of identifiers |
400 | 205106 | maximum number of identifiers exceeded |
Success Response
Field | Type | Description |
---|---|---|
items[].id | integer | submission id |
items[].executing | boolean | indicates whether submission is being executed |
items[].date | string |
date and time of submission creation [yyyy-mm-dd hh:mm:ss TZD] note that server time is used |
items[].compiler | object | submission compiler |
items[].compiler.id | integer | compiler id |
items[].compiler.name | string | compiler name |
items[].compiler.version | object | compiler version |
items[].compiler.version.id | integer | compiler version id |
items[].compiler.version.name | string | compiler version name |
items[].result | object | submission result |
items[].result.status | object | submission status |
items[].result.status.code | integer |
status code see section Submission status |
items[].result.status.name | string | status name |
items[].result.time | float | execution time [seconds] |
items[].result.memory | integer | memory consumed by the program [kilobytes] |
items[].result.signal | integer | signal raised by the program |
items[].result.signal_desc | string | description of the raised signal |
items[].uri | string | link to submission details |
Examples
curl -X GET 'https://<endpoint>/api/v4/submissions?ids=:ids&access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmissions() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmissions(array(2017, 2018));
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.getMulti() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.getMulti([2017, 2018])
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionsIds = [2017, 2018];
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions?ids=' + submissionsIds.join() + '&access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // list of submissions in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
{
"items": [
{
"id": 1,
"executing": false,
"date": "2017-02-24 14:24:21 +00:00",
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 6.3"
}
},
"result": {
"status": {
"code": 15,
"name": "accepted"
},
"time": 0.07,
"memory": 66880,
"signal": 0,
"signal_desc": ""
},
"uri": "https://<endpoint>/api/v4/submissions/1?access_token=<access_token>"
},
{
"id": 2,
"executing": true,
"date": "2017-02-24 14:24:21 +00:00",
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 6.3"
}
},
"result": {
"status": {
"code": 0,
"name": "waiting..."
},
"time": null,
"memory": null,
"signal": null,
"signal_desc": null
},
"uri": "https://<endpoint>/api/v4/submissions/2?access_token=<access_token>"
}
]
}
Returns information about a submission.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | submission id |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | submission not found |
Success Response
Field | Type | Description |
---|---|---|
id | integer | submission id |
executing | boolean | indicates whether submission is being executed |
date | string |
date and time of submission creation [yyyy-mm-dd hh:mm:ss TZD] note that server time is used |
compiler | object | submission compiler |
compiler.id | integer | compiler id |
compiler.name | string | compiler name |
compiler.version | object | compiler version |
compiler.version.id | integer | compiler version id |
compiler.version.name | string | compiler version name |
result | object | submission result |
result.status | object | submission status |
result.status.code | integer |
status code see section Submission status |
result.status.name | string | status name |
result.time | float | execution time [seconds] |
result.memory | integer | memory consumed by the program [kilobytes] |
result.signal | integer | signal raised by the program |
result.signal_desc | string | description of the raised signal |
result.streams | object | submission streams |
result.streams.source | object | source code |
result.streams.source.size | integer | source code length [bytes] |
result.streams.source.uri | string | link to the file with source code |
result.streams.input | object | input data |
result.streams.input.size | integer | size of input data [bytes] |
result.streams.input.uri | string | link to the file with input data |
result.streams.output | object | output data |
result.streams.output.size | integer | size of output data [bytes] |
result.streams.output.uri | string | link to the file with output data |
result.streams.error | object | error data |
result.streams.error.size | integer | size of error data [bytes] |
result.streams.error.uri | string | link to the file with error data |
result.streams.cmpinfo | object | compilation info |
result.streams.cmpinfo.size | integer | size of compilation data [bytes] |
result.streams.cmpinfo.uri | string | link to the file with compilation data |
Examples
curl -X GET 'https://<endpoint>/api/v4/submissions/:id?access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmission() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmission(2017);
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the submission is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Submission does not exist';
}
}
"""
Example presents error handling for submissions.get() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.get(2017)
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the submission is forbidden')
elif e.code == 404:
print('Submission does not exist')
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 2017;
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions/' + submissionId + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(JSON.parse(response.body)); // submission data in JSON
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
}
if (response.statusCode === 403) {
console.log('Access denied');
}
if (response.statusCode === 404) {
console.log('Submision not found');
}
}
}
});
using System;
using System.IO;
using System.Net;
using System.Text;
using System.Collections.Generic;
using System.Collections.Specialized;
namespace csharpexamples {
public class getSubmission {
public static void Main(string[] args) {
// define access parameters
string endpoint = "<endpoint>";
string accessToken = "<access_token>";
// define request parameters
int submissionId = 2017;
try {
WebClient client = new WebClient();
// send request
byte[] responseBytes = client.DownloadData("https://" + endpoint + "/api/v4/submissions/" + submissionId + "?access_token=" + accessToken);
string responseBody = Encoding.UTF8.GetString(responseBytes);
// process response
Console.WriteLine(responseBody);
} catch (WebException exception) {
WebResponse response = exception.Response;
HttpStatusCode statusCode = (((HttpWebResponse) response).StatusCode);
// fetch errors
if (statusCode == HttpStatusCode.Unauthorized) {
Console.WriteLine("Invalid access token");
} else if (statusCode == HttpStatusCode.Forbidden) {
Console.WriteLine("Access denied");
} else if (statusCode == HttpStatusCode.NotFound) {
Console.WriteLine("Submission not found");
}
response.Close();
}
}
}
}
require 'net/http'
require 'uri'
require 'json'
# define access parameters
endpoint = "<endpoint>"
access_token = "<access_token>"
# define request parameters
submission_id = "2017"
# send request
uri = URI.parse("https://" + endpoint + "/api/v4/submissions/" + submission_id + "?access_token=" + access_token)
http = Net::HTTP.new(uri.host, uri.port)
begin
response = http.request(Net::HTTP::Get.new(uri.request_uri))
# process response
case response
when Net::HTTPSuccess
puts JSON.parse(response.body)
when Net::HTTPUnauthorized
puts "Invalid access token"
when Net::HTTPForbidden
puts "Access denied"
when Net::HTTPNotFound
puts "Submission not found"
end
rescue => e
puts "Connection error"
end
Response example
{
"id": 1,
"executing": false,
"date": "2017-02-24 14:24:21 +00:00",
"compiler": {
"id": 1,
"name": "C++",
"version": {
"id": 1,
"name": "gcc 6.3"
}
},
"result": {
"status": {
"code": 15,
"name": "accepted"
},
"time": 0.4,
"memory": 2048,
"signal": 0,
"signal_desc": "",
"streams": {
"source": {
"size": 189,
"uri": "https://<endpoint>/api/v4/submissions/1/source?access_token=<access_token>"
},
"input": {
"size": 56,
"uri": "https://<endpoint>/api/v4/submissions/1/input?access_token=<access_token>"
},
"output": {
"size": 11,
"uri": "https://<endpoint>/api/v4/submissions/1/output?access_token=<access_token>"
},
"error": null,
"cmpinfo": null
}
}
}
Returns raw stream data.
Request parameters
Name | Located in | Type | Description |
---|---|---|---|
access_token |
query | string | access token |
id |
path | integer | submission id |
stream |
path | string |
stream name enum: source, input, output, error or cmpinfo |
Response HTTP Code
HTTP Code | Error Code | Description |
---|---|---|
200 | - | success |
401 | 1 | unauthorized access |
403 | 1002 | access denied |
404 | 1001 | submission not found |
404 | 1101 | stream not found |
400 | 1103 | reading stream failed |
Examples
curl -X GET 'https://<endpoint>/api/v4/submissions/:id/output?access_token=<access_token>'
<?php
/**
* Example presents error handling for getSubmissionStream() API method
*/
use SphereEngine\Api\CompilersClientV4;
use SphereEngine\Api\SphereEngineResponseException;
// require library
require_once('../../../../vendor/autoload.php');
// define access parameters
$accessToken = '<access_token>';
$endpoint = '<endpoint>';
// initialization
$client = new CompilersClientV4($accessToken, $endpoint);
// API usage
try {
$response = $client->getSubmissionStream(2017, 'stdout');
} catch (SphereEngineResponseException $e) {
if ($e->getCode() == 401) {
echo 'Invalid access token';
} elseif ($e->getCode() == 403) {
echo 'Access to the submission is forbidden';
} elseif ($e->getCode() == 404) {
echo 'Non existing resource, error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
} elseif ($e->getCode() == 400) {
echo 'Error code: '.$e->getErrorCode().', details available in the message: ' . $e->getMessage();
}
}
"""
Example presents error handling for submissions.getStream() API method
"""
from sphere_engine import CompilersClientV4
from sphere_engine.exceptions import SphereEngineException
# define access parameters
accessToken = '<access_token>'
endpoint = '<endpoint>'
# initialization
client = CompilersClientV4(accessToken, endpoint)
# API usage
try:
response = client.submissions.getStream(2017, 'stdout')
except SphereEngineException as e:
if e.code == 401:
print('Invalid access token')
elif e.code == 403:
print('Access to the submission is forbidden')
elif e.code == 404:
print('Non existing resource, error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
elif e.code == 400:
print('Error code: ' + str(e.error_code) + ', details available in the message: ' + str(e))
var request = require('request');
// define access parameters
var accessToken = '<access_token>';
var endpoint = '<endpoint>';
// define request parameters
var submissionId = 2017;
var stream = 'output'
// send request
request({
url: 'https://' + endpoint + '/api/v4/submissions/' + submissionId + '/' + stream + '?access_token=' + accessToken,
method: 'GET'
}, function (error, response, body) {
if (error) {
console.log('Connection problem');
}
// process response
if (response) {
if (response.statusCode === 200) {
console.log(response.body); // raw data from selected stream
} else {
if (response.statusCode === 401) {
console.log('Invalid access token');
} else if (response.statusCode === 403) {
console.log('Access denied');
} else if (response.statusCode === 404) {
var body = JSON.parse(response.body);
console.log('Non existing resource, error code: ' + body.error_code + ', details available in the message: ' + body.message)
} else if (response.statusCode === 400) {
var body = JSON.parse(response.body);
console.log('Error code: ' + body.error_code + ', details available in the message: ' + body.message)
}
}
}
});
Response example
Raw data from selected stream.